- Sky
- Blueberry
- Slate
- Blackcurrant
- Watermelon
- Strawberry
- Orange
- Banana
- Apple
- Emerald
- Chocolate
- Charcoal
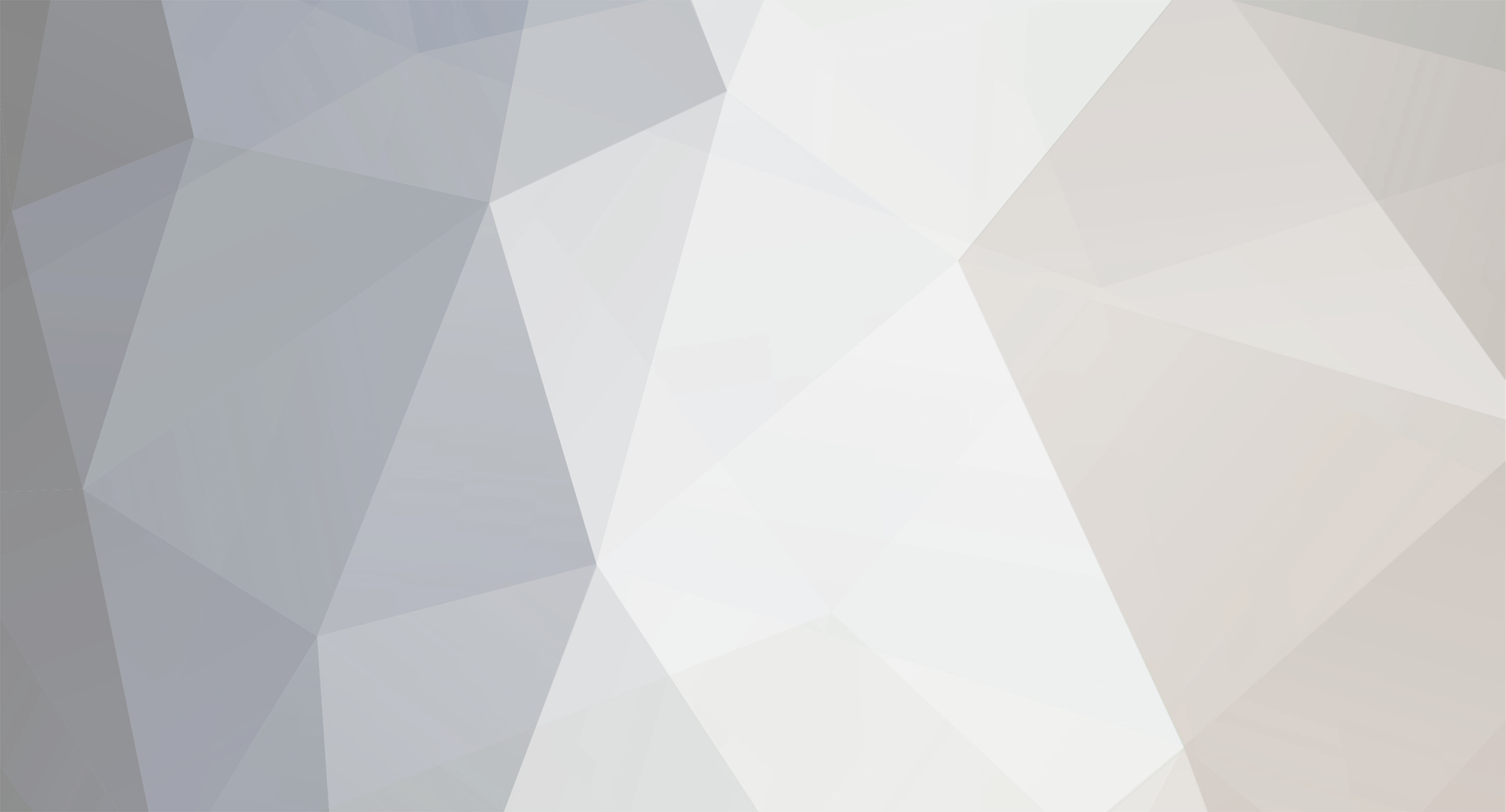
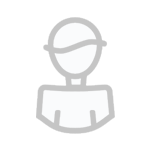
pedrosgali
-
Content Count
42 -
Joined
-
Last visited
-
Days Won
6
Posts posted by pedrosgali
-
-
Hey guys, finally added the library for calling images yourself.
Sorry it took so long but I wrote a new compression method that will save you a lot of RAM, this is done automatically on loading an image. It also required a new render method which is only marginally faster but still a little faster.
Happy painting
-
Thanks man.
I've been asked to start an after school club to teach kids the basics of programming using minecraft, just been looking through my old code to see what I can salvage.
-
Hey guys, been away for a while. I'll post an API for displaying the images as stand alone data later tonight, didn't really think about it at the time lol.
-
robot.turnLeft() is the command, all commands are in camelCase so the first word is not upper case but the first letter of each subsequent word is.
-
Shouldn't it read robot.turnLeft() capitol L
-
-
I tend to use a slightly different method but the above example is perfectly fine, I'll post mine in case you find it easier and partly to show my favourite thing about coding... Mainly that there is never just one right answer.
I do most of my processing in the loop so...
local event = require("event") local keyboard = require("keyboard") local running = true while running do ev, p1, p2, p3, p4, p5 = event.pull(1, _, ev, p1, p2, p3, p4, p5) if ev == "key_down" then local char = keyboard.keys[p3] if char == "w" then --your move forward code block here elseif char == "s" then --your move backward code block here elseif char == "a" then --your move left code block here elseif char == "d" then --your move right code block here elseif char == "q" then running = false end elseif ev == "touch" --do other cool stuff end end
While writing that I thought... What if it went forward like 10 if it received a W instead of a w that would be cool. Then I thought why such an arbitrary number? why not have numerical inputs set the distance? Like this...
local event = require("event") local keyboard = require("keyboard") local distance = 1 local running = true while running do ev, p1, p2, p3, p4, p5 = event.pull(1, _, ev, p1, p2, p3, p4, p5) if ev == "key_down" then local char = keyboard.keys[p3] if tonumber(char) then --Edited to include Sangars change... for ease of copy pasting. if char == 0 then distance = 10 else distance = char end end if char == "w" then for i = 1, distance do --your move forward code block here end elseif char == "s" then --your move backward code block here elseif char == "a" then --your move left code block here elseif char == "d" then --your move right code block here elseif char == "q" then running = false end elseif ev == "touch" --do other cool stuff end end
Haven't used tonumberx in OC yet so not 100% sure that it works but it's like tonumber except it returns boolean according to the Lua reference manual.
That modification should let you use the numbers 0-9 as 1-10, 0 being 10.
Hope this helps.
-
Nickname: Pete
Age: Too old to be playing Minecraft.
Minecraft Name: PedrosGali
IRC nick: pedrosgali -
Yeah, I wrote this before openOS was installable. It was OC 1.2 or something, the /lib folder was read only.
*Edit: Can a moderator delete this thread as it is out of date and may cause confusion to some.
-
-
Actually it's a window manager, no desktop as such. It is literally a front end GUI for openOS.
It does allow multiple 'window' programs to all be running at once and I do plan to add a desktop environment at a later date.
I just can't figure out how to get icons and shortcut without the framerate going down the toilet. Yet.
You're right about the pictures though, I need some screen shots. I'll put some up after work. -
-
In fairness yeah, it should be a local.
-
I tend to opt for a boolian switch for exiting,
running = true
while running do
run()
end
then you have a variable 'running' which can be toggled. Anywhere.
-
I made a step sequencer as my first project for OC, I ran into this problem too.
My solution as yet unmade was to have multiple computers each with a copy of the 'score'waiting for a midi tick from a server.
My code is still in the showcase if you'd like to take a look.
-
Minejaro
0.1
Manjaro style front end for OC.
++ UPDATED ++
Now with text editing!
Minejaro is currently only 5 files:
A library to handle all the window spawning and such.
The screen library must live in your /lib folder and be called screen.lua, it returns many functions that can be called by any window.
you call it like any other library
local scr = require("screen")
local eg = scr:newPane(eg, width, height) --This creates a new 'Pane' that has the following functions attached...
--BASIC FUNCTIONS--
eg:resize(width, height)
--will resize your pane
eg:move(xPos, yPos)
--will move your pane around the screen
eg:center()
--will center your pane on the screen
eg:box(stx, sty, width, height, colour)
--will add a box to your pane making a numbered table called eg.boxTab within.
eg:text(xPos, yPos, bgCol, fgCol, text)
--will add text to your pane.
--NOTE: to clear this text you will need to set eg.printTab to nil and re write your text to it
--OR: know which entry in the table it is (they are numbered in the order you create them.) and nil that (eg.printTab[yourValue] = nil)
eg:centerText(stx, sty, endx, bgCol, fgCol, text)
--will add text centered between stx and endx
eg:render()
--will draw your pane
--DATA READOUT FUNCTIONS--Chat logs etc.
eg:addTextBox(label, xPos, yPos, width, height, bgCol, fgCol)
--will establish a data readout box that you can print to.
--these are brilliant for debug boxes and chat logs. text scrolls if you exceed the box height in lines of text
eg:printText(label, text)
--will print text in the textbox with a matching label.
--BUTTONS--
eg:button(label, stx, sty, width, height, colour, returnValue, textCol)
--will make you a button on screen. establishes eg.buttonTab numbering all buttons as they are created
--textCol defaults to black so unless you need coloured text you can miss it out.
eg:buttonClick(clickX, clickY)
--This goes in your run loop give it the x and y coords of a "touch" event and it will return the buttons returnValue or false if no button was clicked
--TEXT INPUTS--
eg:inputBox(identifier, xPos, yPos, width, height, scale, bgCol, fgCol)
--Adds a text input box to your pane, leave scale as _ if you dont want your box to change size on a resize event.
--Text inputs are stored in the numbered table eg.textInputs
eg:addText(char)
--adds a single character to the currently selected input box,
--WARNING: Using io.read() will lead to horrific screen tear!!! with this API if you want to input text you MUST pull each keystroke
--as an event... there is an example further down VV
eg:textInputClicked(clickX, clickY)
--goes in your run loop again, will return boolien if a box was clicked it becomes selected for the purposes of addText(char)
--IMAGES--
eg:loadImage(label, path, xPos, yPos)
--will load any image made in paintPlus and display it in your pane.
--Thats a basic list of the functions I think people will use most, there are more if you want to look if you have any trouble just post.
--LOADING YOUR OBJECT INTO MINEJARO--
--Minejaro opens a global table on startup called 'st' short for screen table.
--This table holds all the pane data for every open pane.
--Each pane needs an inbuilt function called 'run' which Minejaro will pass all event data to.
--Heres an example:
--now if you are not using Minejaro then just call this function run and loop it following an event.pull with all the same parameters
--and your program will run.
function eg:run(ev, p1, p2, p3, p4, p5)
if ev == "touch" then
if self:clicked(p2, p3) then --Will check if the screen click is within the pane
ret = self:buttonClicked(p2, p3) --Will check for buttons at screen click
if ret == "your buttons returnValue" then
--do your code for that button.
self.needRender = true --set this line if you want minejaro to draw your pane at this point.
return true --This is important so Minejaro knows there was a click and it can stop looking.
end
if self.textInputClicked() then return true end --if you have text inputs then you*ll need this line.
self.grabbed = not self.grabbed --toggles self.grabbed, built in movement sensor for drag and drop.
end
elseif ev == "drag" then
if self.grabbed then --If grabbed is toggled true then
term.clear() --Clear the screen.
self:move(p2, p3) --Move the pane.
self.needRender = true --tell Minejaro to draw the outline of it.
return true --Tell Minejaro its all good.
end
elseif ev == "drop" then
self.grabbed = false --we*re not grabbed any more.
elseif ev == "key_down" then
local char = keyboard.keys[p3]
if char == "enter" then
--do some cool stuff
return true -- Remember to tell Minejaro that it found what it is looking for
end
if keyboard.isShiftDown() then
self:addText(string.upper(keyboard.keys[p3]))
else
self:addText(keyboard.keys[p3])
end
end
end
--I hope that makes sense to you, with that template you*ve got a pane with as many buttons as you
--put in all being checked and executed, it*s a grabbable object that can be moved round the
--screen and can input text into text boxes. There is a lot you can do with that.
--To load your pane into the global filetable (st) it needs an id...
eg.id = #st + 1
--then the last line of your program should read...
st[eg.id] = eg
--Which loads it as a separate entity into the global screen table.
A manager program that allows programs to run.
manager must live in your /bin folder. Run 'manager' to start it.
'konSoul' - a console program.
konSoul is my first draft at a console program, it has a few quirks at the minute.
all prints from programs print to your desktop not the console which is a bug I'm going to fix but is actually kinda fun.
Needs to live in /bin folder.
'Porpoise' - a file browser.
Lives in /bin folder again.
Porpoise is the basic file browser from paintPlus with the addition of a text input box at the top for typing the path.
left clicking will open folders or run programs
right clicking will open folders or open files in edit
'Ate' - A Text Editor
lives in /bin again
It's a text editor, not much to describe.
To those that like pastebin:
Here's the pastebin links
Screen.lua -- Install to /lib/screen.lua
Manager -- Install to /bin/Manager
konSoul -- Install to /bin/konSoul
Browser -- Install to /bin/Browser
Ate -- Install to /bin/Ate
For those that don't I will be putting it on oppm as soon as I can figure out that pesky table.
That's it for now but I'm currently working on a notepad program so you can have multiple text files open at once.
It is possible for you to write your own programs that run in Minejaro, you could have reports and controls from all your different base areas set up as seperate windows. (I know I will.)
I included some brief info on the library in the spoiler above, I'll write a guide here later if anyone has trouble getting programs to run.
-
This idea is awesome, I have no clue about your networking problem however.
I really want to see 4 player pong for this cabinet, each player takes one screen edge and any missing players their edge becomes a wall. So it could serve as 1-4 player pong!
-
Hey man, thanks for the bug report.
As far as I remember the game should only read the HDD when it loads an Image which is often as RAM limits the amount I can keep loaded.
I'll look into events later today, This thing still needs an update but I've got a secret project in the works at the minute.
-
It's a trading game, most things on the screen are buttons to click. Each station has 1 product that you can buy and up to 3 resources you can sell it to operate. Explore and find more stations and AI traders who take all your best trades.
Also there is a close option in the menu.
-
"cd" is the command to switch folders, so "cd SpaceGame0.1.1" then "space".
You may have some trouble if you are not running in max resolution though.
-
I have seen this game crash in many (sometimes spectacular) ways but this is a new one. Well done.
Where are you launching the game from? did you navigate to the SpaceGame folder and launch from there? or did you do "SpaceGame/space" to launch?
That would cause a crash sort of like this as the game would not find the right working directory and thus none of the assets.
Hope this helps, and thank you for the bug report.
Also this is due for an update soon, working on assets at the minute but in my copy Shipyards are in so you can buy multiple ships and there are a few pieces of equipment to buy at Equipment docks. More Equipment is pending.
Sorry for the delay in updating but I got a 3D printer last week and have been tinkering with that instead of coding
-
You could try checking on the keypress if shift is down then use string.upper(char)
Like this:
if keyboard.isShiftDown() then --Shift pressed. char = string.upper(char) end print(char)
Hope this helps
--EDIT:
After reading your post again it looks like your code will detect the pressing of shift as a keypress and print the result anyway. You'll have to account for that.
Maybe like this:
while true do ev, p1, p2, p3, p4, p5 = event.pull(.01, _, ev, p1, p2, p3, p4, p5) if ev == "key_down" then local char = keyboard.keys[p3] if char == "left_shift" or char == "right_shift" then else if keyboard.isShiftDown() then --Shift pressed. char = string.upper(char) end print(char) end end end
I think p3 is the charachter, I'm not sure and I don't really want to check right now.
Let me know if you have any trouble.
-
Wasn't the chat log scanner from another mod? by richard G perhaps? I had something like this set up when I was using CC and extra peripherals but as far as I know there is no peripheral that serves this function yet in OC. I might be wrong about that... I hope I am.
If I am wrong someone please let me know so I can get the IRC feed to come up in my in game chat log
-
type "edit <filename>". Happy coding
Paint Plus. ++Now with a library to load the pictures!++
in Programs
Posted
Thanks man, I used the library from this to make Minejaro. It's a front end GUI for openOS, if you like the style of this you should check it out. It needs looking at again really but I rarely have time these days.