- Sky
- Blueberry
- Slate
- Blackcurrant
- Watermelon
- Strawberry
- Orange
- Banana
- Apple
- Emerald
- Chocolate
- Charcoal
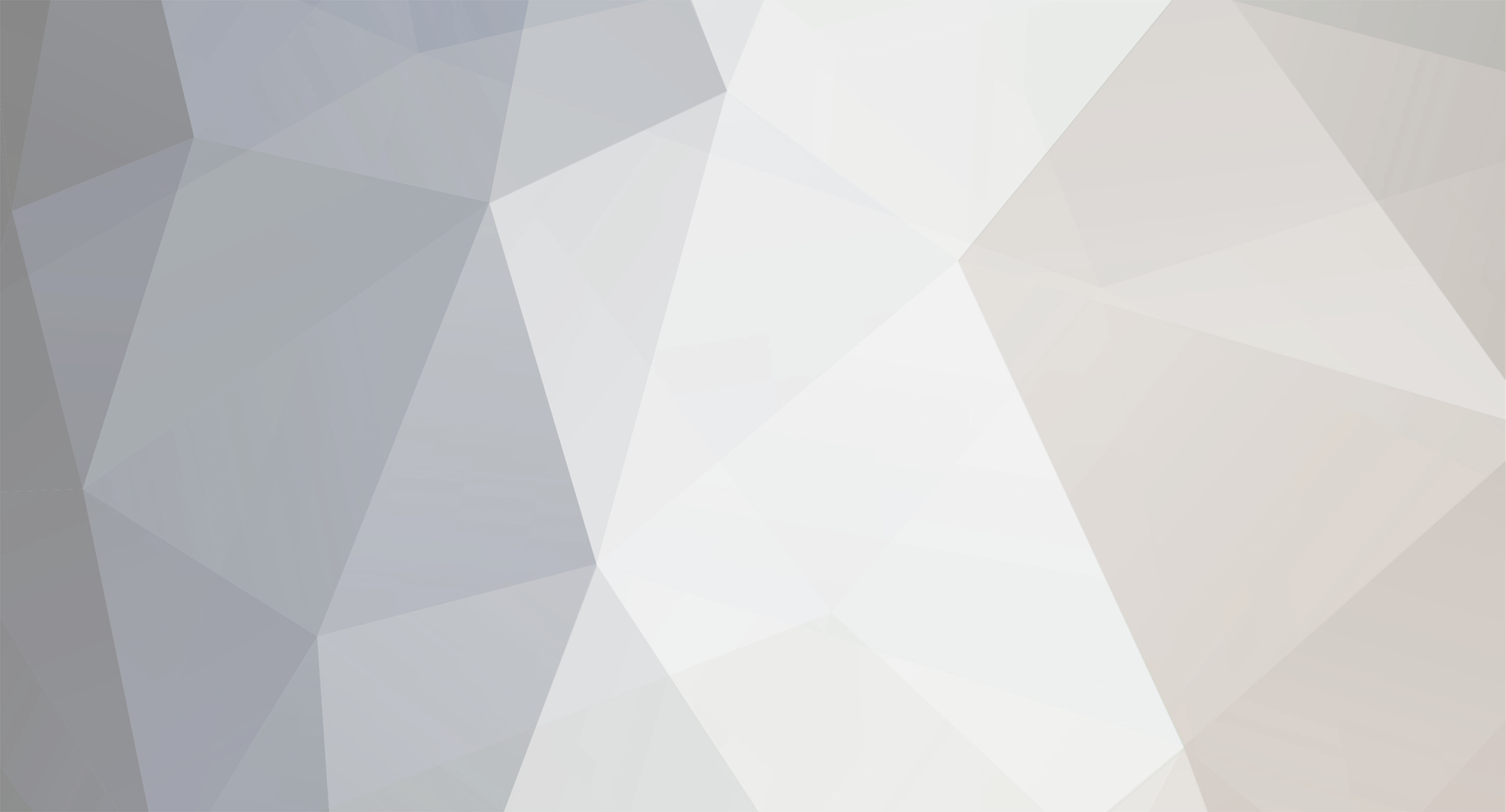
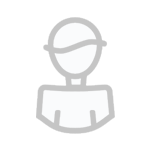
Tag365
-
Content Count
15 -
Joined
-
Last visited
-
Days Won
2
Posts posted by Tag365
-
-
Firstly, the robot must have an inventory upgrade so it can have an inventory so it can place and obtain blocks. The script makes the robot go up to eight blocks above the ground to take down the tree, so you may also need a hover upgrade which increases the amount of blocks the robot can go above from the ground. To get it to work you must have put saplings in the first inventory slot of the robot. You should also have an axe as the tool to claim the wood obviously, make sure you put the axe where the wrench icon is in the inventory. Also, make sure you have a flat surface of dirt so the robot can move properly without being blocked and actually place the saplings. I believe that is all the stuff you need to get it to work.
-
A basic tree farm for a robot. By default it will start a 6x6 tree farm but you can configure the amount of trees in the grid as well as how far each tree will be from each other.
Firstly, the robot must have an inventory upgrade so it can have an inventory so it can place and obtain blocks. The script makes the robot go up to eight blocks above the ground to take down the tree, so you may also need a hover upgrade which increases the amount of blocks the robot can go above from the ground. To get the script to plant the trees properly you must have put saplings in the first inventory slot of the robot. You should also have an axe as the tool to claim the wood faster obviously, make sure you put the axe where the wrench icon is in the inventory. Also, make sure you have a flat surface of dirt so the robot can move properly without being blocked and actually place the saplings.
To get the script on your computer, if you have an internet component installed execute "pastebin get cg1ieKUi autorun.lua" and the required autorun script will be downloaded, or if you are the server host get the script at http://www.pastebin.com/raw/cg1ieKUi and add the script to the root of the filesystem that hosts the operating system.
local robot = require("robot") print("Robot tree farm started") -- Select the first slot, which is supposed to have a sapling. robot.select(1) -- Change these to change the tree farm grid size or the distance between each tree in the grid. local treesX = 6 local treesZ = 6 local distanceBetweenTrees = 5 -- Goes forward eventually, no matter if something is blocking the path at the moment. local function GoForward() while true do local movedSuccessfuly = robot.forward() if movedSuccessfuly then break end end end -- Goes down eventually, no matter if something is blocking the path at the moment. local function GoDown() while true do local movedSuccessfuly = robot.down() if movedSuccessfuly then break end end end -- Checks for a tree local function CheckForTree() -- Check for a block if robot.detect() then robot.up() -- Attempt to detect a block above which will determine if the tree has grown. local blockFound = robot.detect() robot.down() -- Check tree has grown and if so then go up. if blockFound then for blocksToMoveUp = 1, 8 do -- Destroy the wood in front of the robot robot.swing() -- Check if there is a block above and if so then destroy the block. if robot.detectUp() then robot.swingUp() end -- Go up robot.up() end -- Move back down again for blocksToMoveDown = 1, 8 do GoDown() end -- Suck up stuff and go forward robot.suck() robot.suckUp() robot.suckDown() GoForward() -- Suck up stuff for rotation = 1, 4 do robot.turnLeft() robot.suck() end -- Go back robot.turnAround() GoForward() robot.turnAround() -- Place the new sapling here robot.place() end else -- There is no block here, place the sapling robot.place() end end -- Scans a row of trees. local function CheckRowOfTrees() -- Check for trees in the X row. for treeX = 1, treesX do CheckForTree() -- If this isn't the last tree in the row then move to the next tree in the row. if treeX < treesX then robot.turnRight() for blocksToMove = 1, distanceBetweenTrees do GoForward() robot.suck() end robot.turnLeft() end end -- Go back to the first tree in the row. robot.turnLeft() for blocksToMove = 1, distanceBetweenTrees*(treesX - 1) do GoForward() end robot.turnRight() end -- Do the complete cycle. while true do -- Go to each X row in the grid. for treeZ = 1, treesZ do CheckRowOfTrees() -- If this isn't the last X row in the grid then go to the next X row in the grid. if treeZ < treesZ then robot.turnRight() GoForward() robot.turnLeft() for blocksToMove = 1, distanceBetweenTrees do GoForward() robot.suck() end robot.turnLeft() GoForward() robot.turnRight() end end -- Go back to the starting position. robot.turnRight() GoForward() robot.turnRight() for blocksToMove = 1, distanceBetweenTrees*(treesZ - 1) do GoForward() robot.suck() end robot.turnRight() GoForward() robot.turnRight() -- Sleep for five seconds. os.sleep(5) end
-
Here is a Lua script for a basic 6x6 tree farm.
Try inspecting the script to figure out how it works and modifying the script to your liking.
local robot = require("robot") print("Robot tree farm started") -- Select the first slot, which is supposed to have a sapling. robot.select(1) -- Change these to change the tree farm grid size or the distance between each tree in the grid. local treesX = 6 local treesZ = 6 local distanceBetweenTrees = 5 -- Goes forward eventually, no matter if something is blocking the path at the moment. local function GoForward() while true do local movedSuccessfuly = robot.forward() if movedSuccessfuly then break end end end -- Goes down eventually, no matter if something is blocking the path at the moment. local function GoDown() while true do local movedSuccessfuly = robot.down() if movedSuccessfuly then break end end end -- Checks for a tree local function CheckForTree() -- Check for a block if robot.detect() then robot.up() -- Attempt to detect a block above which will determine if the tree has grown. local blockFound = robot.detect() robot.down() -- Check tree has grown and if so then go up. if blockFound then for blocksToMoveUp = 1, 8 do -- Destroy the wood in front of the robot robot.swing() -- Check if there is a block above and if so then destroy the block. if robot.detectUp() then robot.swingUp() end -- Go up robot.up() end -- Move back down again for blocksToMoveDown = 1, 8 do GoDown() end -- Suck up stuff and go forward robot.suck() robot.suckUp() robot.suckDown() GoForward() -- Suck up stuff for rotation = 1, 4 do robot.turnLeft() robot.suck() end -- Go back robot.turnAround() GoForward() robot.turnAround() -- Place the new sapling here robot.place() end else -- There is no block here, place the sapling robot.place() end end -- Scans a row of trees. local function CheckRowOfTrees() -- Check for trees in the X row. for treeX = 1, treesX do CheckForTree() -- If this isn't the last tree in the row then move to the next tree in the row. if treeX < treesX then robot.turnRight() for blocksToMove = 1, distanceBetweenTrees do GoForward() robot.suck() end robot.turnLeft() end end -- Go back to the first tree in the row. robot.turnLeft() for blocksToMove = 1, distanceBetweenTrees*(treesX - 1) do GoForward() end robot.turnRight() end -- Do the complete cycle. while true do -- Go to each X row in the grid. for treeZ = 1, treesZ do CheckRowOfTrees() -- If this isn't the last X row in the grid then go to the next X row in the grid. if treeZ < treesZ then robot.turnRight() GoForward() robot.turnLeft() for blocksToMove = 1, distanceBetweenTrees do GoForward() robot.suck() end robot.turnLeft() GoForward() robot.turnRight() end end -- Go back to the starting position. robot.turnRight() GoForward() robot.turnRight() for blocksToMove = 1, distanceBetweenTrees*(treesZ - 1) do GoForward() robot.suck() end robot.turnRight() GoForward() robot.turnRight() -- Sleep for five seconds. os.sleep(5) end
-
On 5/18/2016 at 10:45 PM, danman1354 said:
Every time I write a file to the host i have to reset it before any computer on the network can see it in the srv directory, including the pc that wrote it.
Evewn when I get it to show up when I ls, if i open (edit) it, the file is always blank.
Then if i save the file (test.txt), when i open and ls the srv directory on another pc, it will show test.txt and test.txt~, both being empty.
What am I doing wrong?For anyone that says that the files you create from the client are blank, this issue is caused by a change of the filesystem handling in an update to the OpenComputer mod, where file handles are not numbers anymore. I already made an updated version which is compatible with the updated file handles and fixes the problem by using numbers for file handle identification. The updated version to fix the issue has now been published. Update the script on the Host, then test to see if that fixed your issue. If not then report the issue you are having.
-
On 2/4/2017 at 0:41 PM, Sukasa said:
I also cannot get this entirely to work; while the system boots properly and I can create folders / list their contents, the moment I try to work with any files, it falls over. doing something like `cd /srv` and `edit testfile` will seem to work, but the moment I try to save in edit, it throws an error:
bad argument #1 (string expected, got nil):
stack traceback:
[C]: in function 'error'
machine:631: in function <machine:628>
(...tail calls...)
/bin/edit.lua:117: in upvalue 'setSsatus'
/bin/edit.lua:527: in local 'handler'
/bin/edit.lua:583: in local 'onKeyDown'
...On the server's side, I have attached its output log
After that, I attempted to run the command `echo "test" >> test`, and got another error. I have attached that as well
This is caused by a change of the filesystem handling in an update to the OpenComputer mod, where file handles are not numbers anymore. I already made an updated version which is compatible with the updated file handles and fixes the problem by using numbers for file handle identification. However I have not published the updated version yet and I still have the files on my old computer. So wait for the updated version to be published.
The updated version to fix the issue has now been published. Test the updated version now to see if that fixed your issue.
-
I'm amazed by the picture quality as seen in these screenshots. Next step is to convert PNG files to that format. I have an API that allows obtaining color information from PNG image files.
-
As I said it is not designed to handle multiple Hosts running, as it doesn't favor any Host on the network, the first to respond is the one that is used.
-
It is not designed to handle multiple Hosts being online and on the same network. I have not tested it yet, so it may or may not work correctly.
-
In order for me to fix it, you need to tell me what is the error message, or if it does not throw an error, what is happening. Also, you need to give me information on how to get version 1.6 of OpenComputers.
-
The component must have a type of "modem" or "tunnel" in the computer or server. Network cards and Wireless Network cards have a type of "modem", while Linked cards have a type of "tunnel". Therefore, you can use a Network card, Wireless Network card, or Linked card to connect to the Host computer. The other components required are the filesystems you want in the Host's RAID array.
-
Do you plan on using this?
-
This is a remote RAID where you can store and access data from across the network. Filesystems will be detected by the autorun script and included in the array. You can read and write to the filesystem remotely.
-
Using this program, you can host an array filesystem over a network. Set up is as simple as installing an autorun.lua script on the computer or server that hosts the ServerFS filesystem, and installing a boot script on the clients that will access the ServerFS filesystem.
How it works:
The "Host", the computer or server hosting the ServerFS fileserver, runs an autorun script which will allocate all non-primary filesystem components to a single virtual "ServerFS" component. It then receives messages over the network and when a message is received relating to the ServerFS the Host will then respond to the sender with a message with data related to the method it was trying to call.
A "Client", a computer or server connecting to the ServerFS Host, has a boot script installed which runs at startup and sets up a virtual component which functions like a normal filesystem component. Calling one of the virtual component's methods will cause the Client to send messages to the Host computer and wait for the Host to respond. The boot script also automatically mounts the virtual filesystem component to the "srv" directory.
Requirements:
The component must have a type of "modem" or "tunnel" in the computer or server. Network cards and Wireless Network cards have a type of "modem", while Linked cards have a type of "tunnel". Therefore, you can use a Network card, Wireless Network card, or Linked card to connect to the Host computer. The other components required are the filesystems you want in the Host's RAID array.
You must also have at least one filesystem attached to the Host computer.
How to set up ServerFS:
The "Host", the computer or server hosting the ServerFS fileserver.
Step 1: Install a modem or tunnel component (a Network card or Linked card will work for this, as each card acts as a modem or tunnel component respectively) to the Host computer or server, then install any filesystem components you want in the array.
Step 2: Get the autorun.lua script on to the Host. If the Host has an internet component installed execute "pastebin get cg1ieKUi /autorun.lua" and the required autorun script will be downloaded, or if you are the server host get the script at http://www.pastebin.com/raw/cg1ieKUi and add the script to the root of the filesystem that hosts the operating system.
Step 3: Run the autorun.lua script. The autorun script will allocate all non-primary filesystem components to a virtual filesystem array, and then will host the ServerFS functionality.
The "Clients", or computers connecting to the ServerFS fileserver served by the Host.
Step 1: Install a modem or tunnel component to the Client (a Network card, Wireless network card, or Linked card will work), then connect the Client to the Host. Do not connect it to the Host directly if a large amount of components are inserted to the Host, as this can cause the Client to stop and show a "Too many components connected" error.
Step 2: Install the boot script to the Client's boot filesystem. If the Client has an internet component installed execute "pastebin get HAgcbg41 /boot/98_serverfilesystem.lua", or if you are the server host get the script at http://www.pastebin.com/raw/HAgcbg41 and add the script to the path of "/boot/98_serverfilesystem.lua" on the filesystem that hosts the operating system.
Step 3: Reboot the computer. On startup a "srv" directory will be mounted.
Additional Information:
Please note that accessing functions of the server filesystem is noticeably slower than accessing the other filesystems on your network because the Host has to receive the request of the client from the network (which adds a delay that can be reduced by adding a CPU or APU to the relay if there is any), then process the request, and then send the processed information to the client. Don't expect it to be as fast as accessing functions of a filesystem attached to your computer.
If the network has relays connected, if too many requests are sent at in to short a timespan this can cause requests to be dropped by the relay and cause problems.
You should shut down the Host computer before adding or removing filesystems from or to the Host computer, as not doing so can cause issues where the server will attempt to access filesystems that are not attached to the computer.
For anyone that says that the files you create from the client are blank, this issue is caused by a change of the filesystem handling in an update to the OpenComputer mod, where file handles are not numbers anymore. I already made an updated version which is compatible with the updated handling of file handles and fixes the error by using numbers for file handle identification. The updated version to fix the issue has now been published. Update the script on the Host, then test to see if that fixed your issue. If not then report the issue you are having (post the issue again and that you updated the script if the same issue occurs).
Download:
The hosting autorun.lua script is at http://www.pastebin.com/cg1ieKUi
The 98_serverfilesystem.lua script to add in the boot directory of your clients is at http://pastebin.com/HAgcbg41
-
I know that this is very basic. I'm not used to OpenComputers at all, so don't expect much other than the fact that you can download a script from a server hosted by somebody else.
Here is the textutils API script source:
local textutils = {} local g_tLuaKeywords = { [ "and" ] = true, [ "break" ] = true, [ "do" ] = true, [ "else" ] = true, [ "elseif" ] = true, [ "end" ] = true, [ "false" ] = true, [ "for" ] = true, [ "function" ] = true, [ "if" ] = true, [ "in" ] = true, [ "local" ] = true, [ "nil" ] = true, [ "not" ] = true, [ "or" ] = true, [ "repeat" ] = true, [ "return" ] = true, [ "then" ] = true, [ "true" ] = true, [ "until" ] = true, [ "while" ] = true, } local function serializeImpl( t, tTracking, sIndent ) local sType = type(t) if sType == "table" then if tTracking[t] ~= nil then error( "Cannot serialize table with recursive entries", 0 ) end tTracking[t] = true if next(t) == nil then -- Empty tables are simple return "{}" else -- Other tables take more work local sResult = "{\n" local sSubIndent = sIndent .. " " local tSeen = {} for k,v in ipairs(t) do tSeen[k] = true sResult = sResult .. sSubIndent .. serializeImpl( v, tTracking, sSubIndent ) .. ",\n" end for k,v in pairs(t) do if not tSeen[k] then local sEntry if type(k) == "string" and not g_tLuaKeywords[k] and string.match( k, "^[%a_][%a%d_]*$" ) then sEntry = k .. " = " .. serializeImpl( v, tTracking, sSubIndent ) .. ",\n" else sEntry = "[ " .. serializeImpl( k, tTracking, sSubIndent ) .. " ] = " .. serializeImpl( v, tTracking, sSubIndent ) .. ",\n" end sResult = sResult .. sSubIndent .. sEntry end end sResult = sResult .. sIndent .. "}" return sResult end elseif sType == "string" then return string.format( "%q", t ) elseif sType == "number" or sType == "boolean" or sType == "nil" then return tostring(t) else error( "Cannot serialize type "..sType, 0 ) end end EMPTY_ARRAY = {} local function serializeJSONImpl( t, tTracking ) local sType = type(t) if t == EMPTY_ARRAY then return "[]" elseif sType == "table" then if tTracking[t] ~= nil then error( "Cannot serialize table with recursive entries", 0 ) end tTracking[t] = true if next(t) == nil then -- Empty tables are simple return "{}" else -- Other tables take more work local sObjectResult = "{" local sArrayResult = "[" local nObjectSize = 0 local nArraySize = 0 for k,v in pairs(t) do if type(k) == "string" then local sEntry = serializeJSONImpl( k, tTracking ) .. ":" .. serializeJSONImpl( v, tTracking ) if nObjectSize == 0 then sObjectResult = sObjectResult .. sEntry else sObjectResult = sObjectResult .. "," .. sEntry end nObjectSize = nObjectSize + 1 end end for n,v in ipairs(t) do local sEntry = serializeJSONImpl( v, tTracking ) if nArraySize == 0 then sArrayResult = sArrayResult .. sEntry else sArrayResult = sArrayResult .. "," .. sEntry end nArraySize = nArraySize + 1 end sObjectResult = sObjectResult .. "}" sArrayResult = sArrayResult .. "]" if nObjectSize > 0 or nArraySize == 0 then return sObjectResult else return sArrayResult end end elseif sType == "string" then return string.format( "%q", t ) elseif sType == "number" or sType == "boolean" then return tostring(t) else error( "Cannot serialize type "..sType, 0 ) end end function textutils.serialize( t ) local tTracking = {} return serializeImpl( t, tTracking, "" ) end function textutils.unserialize( s ) local func = load( "return "..s, "unserialize" ) if func then local ok, result = pcall( func ) if ok then return result end end return nil end function textutils.serializeJSON( t ) local tTracking = {} return serializeJSONImpl( t, tTracking ) end function textutils.urlEncode( str ) if str then str = string.gsub(str, "\n", "\r\n") str = string.gsub(str, "([^%w ])", function(c) return string.format("%%%02X", string.byte(c)) end ) str = string.gsub(str, " ", "+") end return str end -- GB versions serialise = serialize unserialise = unserialize serialiseJSON = serializeJSON return textutils
Here is the actual script source:local internet = require("internet") local textutils = require("textutils") local fs = require("filesystem") local c = require("computer") local ccsysurl = "https://ccsystems.dannysmc.com/ccsystems.php" local str = "" function freeMemory() local result = 0 for i = 1, 10 do result = math.max(result, c.freeMemory()) os.sleep(0) end return result end function download(url, post) local str = "" for chunk in internet.request(url, post) do str = str..chunk end return str end print("Loading application list.") local str = download(ccsysurl, "ccsys="..textutils.urlEncode(tostring("appstore")).."&".."cccmd="..textutils.urlEncode(tostring("list"))) local appslist = textutils.unserialize(str) str = nil freeMemory() for k, v in ipairs(appslist) do print(k..":"..appslist[k][2]) end print("Type in the number of the application you want to download.") local str = download(appslist[tonumber(io.read()) or 150][6]) print("Where do you want to save to?") local file = fs.open(io.read(), "w") file:write(str) file:close() print("Done saving.")
ServerFS - Host a filesystem over the network.
in Programs
Posted
I fixed the ServerFS host script to actually use the tunnel component if available (not sure if I already added this feature). This means you can now connect a far away computer to the Host and access the ServerFS from anywhere. You should now update the host ServerFS autorun script to add the tunnel functionality.