- Sky
- Blueberry
- Slate
- Blackcurrant
- Watermelon
- Strawberry
- Orange
- Banana
- Apple
- Emerald
- Chocolate
- Charcoal
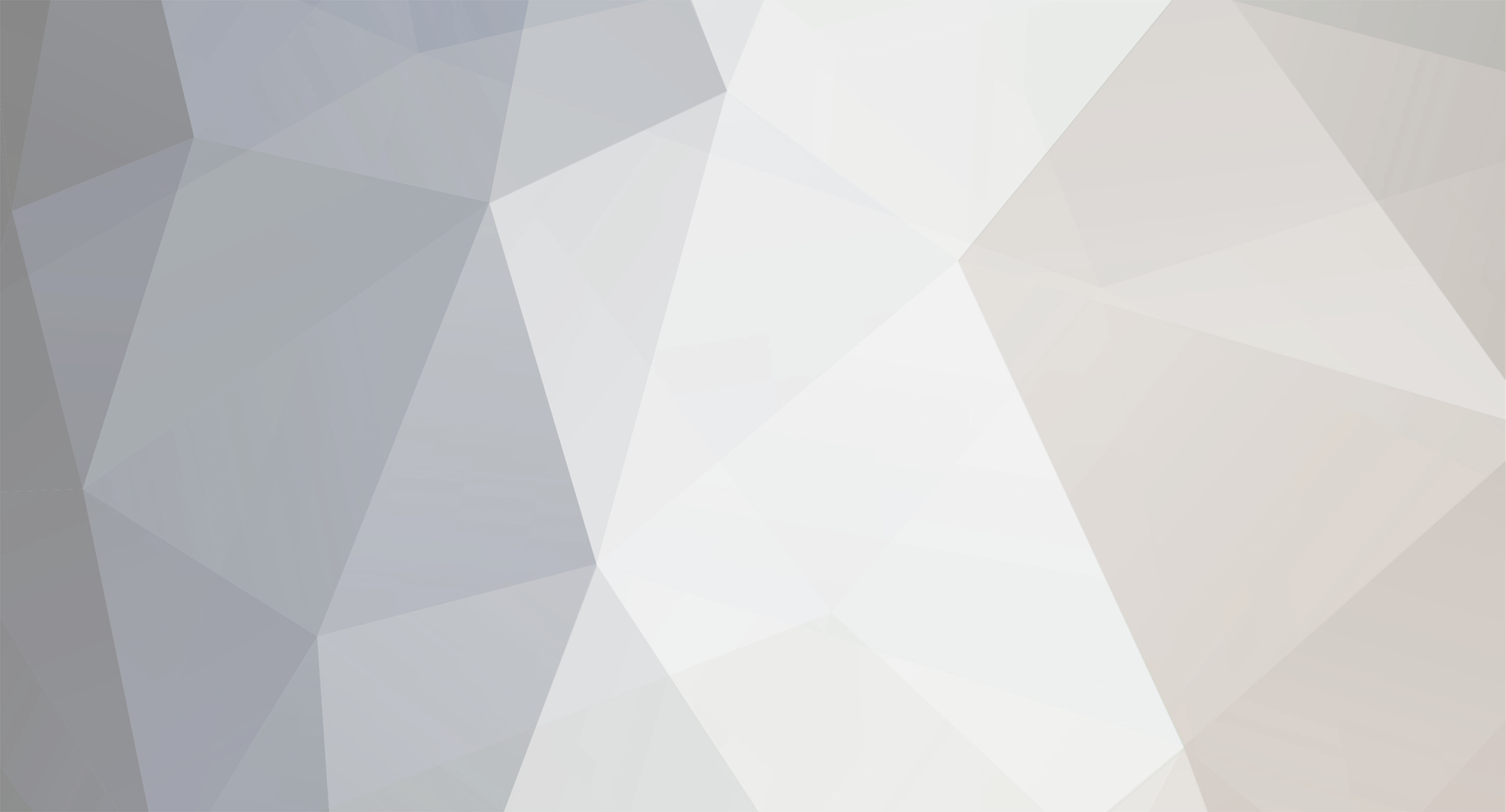
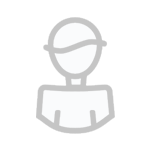
Gorzoid
-
Content Count
111 -
Joined
-
Last visited
-
Days Won
22
Posts posted by Gorzoid
-
-
Shift right click with scrench, but common you could have just googled that.
-
Yeah fixed em both just make another eeprom and put the new code in it and craft it with drone again. I edited my first post with updated code.
-
Updated original code so it will stop to empty the inventory if inventory is full then continue where it left off. It also only ever dropped off the first slot so that would obviously be a big issue in a farm with over 32 plant crops, and 43x43 is alot bigger than that.
-
Wow your farm is that big? If you only have one inventory upgrade then adding another should be a quick fix, but I'll try mess with it so it checks it's inventory after each iteration
-
It says on screen... Ctrl+s to save ctrl+w to close
-
You need to put the code into an eeprom and then the eeprom into the drone, either when your assembling it, or after by putting the drone and the eeprom into a crafting bench.
To put the code into the eeprom, start up a computer as normal, when it has fully loaded, swap out the lua eeprom for a blank one. Then type "edit /dev/eeprom" and copy paste the code into the editor via the Insert key. Then save the code and exit, the eeprom will now have the code. Craft it with the drone to insert it.
-
This was a fun challenge, tried to make it simple, with almost no need to change the code while also being complex enough to expand into big farms. I made this with sugarcane in mind because I am pretty sure they work exactly the same.
local WAIT_TIME = 60 -- time to wait between cycles local drone = component.proxy(component.list("drone")()) local nav = component.proxy(component.list("navigation")()) local vector vector = { __add = function(vec1,vec2) local vec3 = vector {} for i = 1,#vec1 do vec3[i] = vec1[i] + vec2[i] end return vec3 end, __sub = function(vec1,vec2) local vec3 = vector {} for i = 1,#vec1 do vec3[i] = vec1[i] - vec2[i] end return vec3 end, __unm = function(vec1) local vec2 = vector {} for i = 1,#vec1 do vec2[i] = vec1[i] end return vec2 end, unpack = function(tbl) return table.unpack(tbl) end } vector.__index = vector setmetatable(vector, { __call = function(mt,arg1,...) return setmetatable(type(arg1) == "table" and arg1 or {arg1,...},vector) end } ) local plants = {} local charger = vector {0,0,0} local chest = charger do local list = nav.findWaypoints(16) -- Change to increase waypoint search radius, probably uses more power idk for i = 1,#list do local wp = list[i] local direction,length = wp.label:match("^plant:([2-5]):([0-9]+)$") if direction then direction = tonumber(direction) local axis,negate = direction > 3 and 1 or 3, (direction % 2)*2 - 1 for i = 0,negate*(length-1),negate do local pos = {table.unpack(wp.position)} pos[axis] = pos[axis] + i pos[2] = pos[2] + 3 -- Crop height plants[#plants+1] = vector(pos) end end if wp.label:find"chest" then chest = vector(wp.position) end if wp.label:find"charger" then WAIT_TIME = tonumber(wp.label:match("%[([0-9]+)%]")) or WAIT_TIME -- if charger waypoint label contains [number] the waittime will become that number charger = vector(wp.position) end end end local pos = vector {0,0,0} local function move(vec,halt) drone.move(vec:unpack()) if(halt) then local speed = drone.getVelocity() while(true) do computer.pullSignal(0.1) local tmpspeed = drone.getVelocity() if tmpspeed < speed and tmpspeed < 2 then break end speed = tmpspeed end computer.pullSignal(0.5) end pos = pos + vec end local invSize = drone.inventorySize() local currSlot = 1 while true do for i = 1,#plants do move(plants[i] - pos,true) if drone.detect(0) then drone.swing(0) move(vector{0,-1,0},true) drone.swing(0) end if drone.count(currSlot) == 64 then currSlot = currSlot + 1 if currSlot > invSize then move(chest - pos,true) for i = 1,invSize do drone.select(i) drone.drop(0) end currSlot = 1 end end end move(chest - pos,true) for i = 1,currSlot do drone.select(i) drone.drop(0) end currSlot = 1 move(charger - pos) computer.pullSignal(WAIT_TIME) end
If you want to set up a farm you need atleast these two things:
- A drone with a navigation upgrade and atleast one inventory upgrade.
- A waypoint block to mark out where the crops are.
The waypoint labels are what configure the drone, when it starts up it will search for all waypoints in a 16 block radius. There are three tags for the waypoint labels: plant, chest and charger. chest and/or charger are not required if your drone starts up above the chest and/or beside a charging face of the charger, look at the first attached image as an example. If you don't have the drone spawning on one of them you will need a waypoint to mark out where it is, using the label chest or charger. The plant waypoint is a bit more complicated, it uses a format of:
plant:direction:length
Where direction is the sides index that the line of crops extends and length is the number of crops in that direction. Use the sides api to get the correct direction. As an example my farm had 3 sugarcane crops extending south/positive z direction. So my label, was plant:3:3. You can see in the second picture what that looked like.
Hope this works, I actually never knew drones could break/place blocks until now.
-
On 2/19/2017 at 9:02 PM, Michiyo said:
For a computer you have to do
local component = require("component")
In the lua interpreter you don't have to require stuff like you do in scripts
For a microcontroller... well you don't get require there so you'll have to do it lower level and I'm not sure how that works
In eeprom code the component table is never removed from global table so it's fine to use, and his original code should work on a microcontroller since he doesn't use any of the component api additions added by openos.
-
Screenshots would be nice. A suggestion would be to modify the case and screen models. Btw what you mean "acid colour"
-
Find the green disk named OpenOS in the creative menu and use that instead of the blank disk
-
computer.pushSignal does exactly that, call it with arguments in the same order as returned by event.pull
-
Nice work, I think the largest tape drive can store a few MB so you can store quite alot with this, albeit very slowly.
Suggestions:
- "if var == true then" is the exact same as "if var then", just as "if var == false then" is the same as "if not var then"
- You may want to try mount this file to a /dev/tape_drive file. This would mean you could do let's say "cp /dev/tape_drive /home/myfile.txt" to read from tapedrive, or "cp /home/myfile.txt /dev/tape_drive" to write to tapedrive. Check out OpenOS' devfs.lua for info on making fake files.
-
I never use the ingame editor, if your program is small enough you can (under 1024 chars) you can just copy and paste with insert key, otherwise you may want to use a webservice like pastebin to upload your program and download it with an internet card.
Also this is in the wrong section, tutorials is meant for OP to give info, not ask for it, use support subforum next time.
-
sets the environment variable PATH to first argument. PATH is a ; divided list of paths that the shell uses to search for a program when you type a command. basically the same as in most operating systems these days
Also also: this is in the wrong section, tutorials is meant for OP to give info, not ask for it, use support subforum next time.
-
Solved, looks like the github's instructions is outdated, works fine if I use compile "li.cil.oc:OpenComputers:MC1.7.10-1.6.+:dev" instead of compile "li.cil.oc:OpenComputers:MC1.7.10-1.6.+:api" in build.gradle and remove the dev build from mods folder. Thanks Michiyo for helping me on IRC.
-
When I launch Minecraft with OpenComputers dev in eclipse OC spits alot of errors but does not crash, when I go into a world all items don't seem to work (can't put into computer).
Some of the errors include:
[18:39:50] [Client thread/INFO] [OpenComputers/OpenComputers]: Registering new assembler template 'Tablet (Creative)' from mod OpenComputers. [18:39:50] [Client thread/WARN] [OpenComputers/OpenComputers]: Failed registering assembler template. java.lang.ClassCastException: interface li.cil.oc.api.internal.Tablet
Entire log: http://pastebin.com/YEteSicq
I don't think it is my mods doing, since even if I comment out the 2 lines of code that run it will still break. If I build the mod it works fine with regular universal build. I didn't make an issue on github because I am not sure if this is just me messing up or if it's a problem with the dev build since I tried it on 3 versions.
-
Check out CC forums, sadly even though alot of them use OpenComputers, they don't post their server on this forum too, while your at it, you may want to ask the owner if they can post it over here too.
-
Updated OP.
-
So really you just want an OC emulator? There is one or two on the forums.
--Edit:
If your talking about making a full baremetal OS that acts like OpenOS it would be very difficult especially if you don't know any low level languages.
-
Map<String, Callback> li.cil.oc.api.machine.Machine.methods(Object value); where value is the component
-
this is probably an issue that needs to be fixed in the code, we need a way to choose how adjacent components get connected
-
In multiplayer it will check if the owner is allowed to build in that area iirc. Or the server may have disabled robot block breaking all together.
-
not sure if OpenOS allows advanced redirecting but you can try
program.lua 2> errorlog.txt
-
I don't think so, I had this problem before and I just decided to make a quick program that pulled the component_added signal and logged it to a file in order, then I would just load the file in the program and use it there
Drone Harvesting program request
in Requests
Posted
You didn't put the code in, you just put a regular lua eeprom in it. Please read the instructions I already gave on how to install the code.