- Sky
- Blueberry
- Slate
- Blackcurrant
- Watermelon
- Strawberry
- Orange
- Banana
- Apple
- Emerald
- Chocolate
- Charcoal
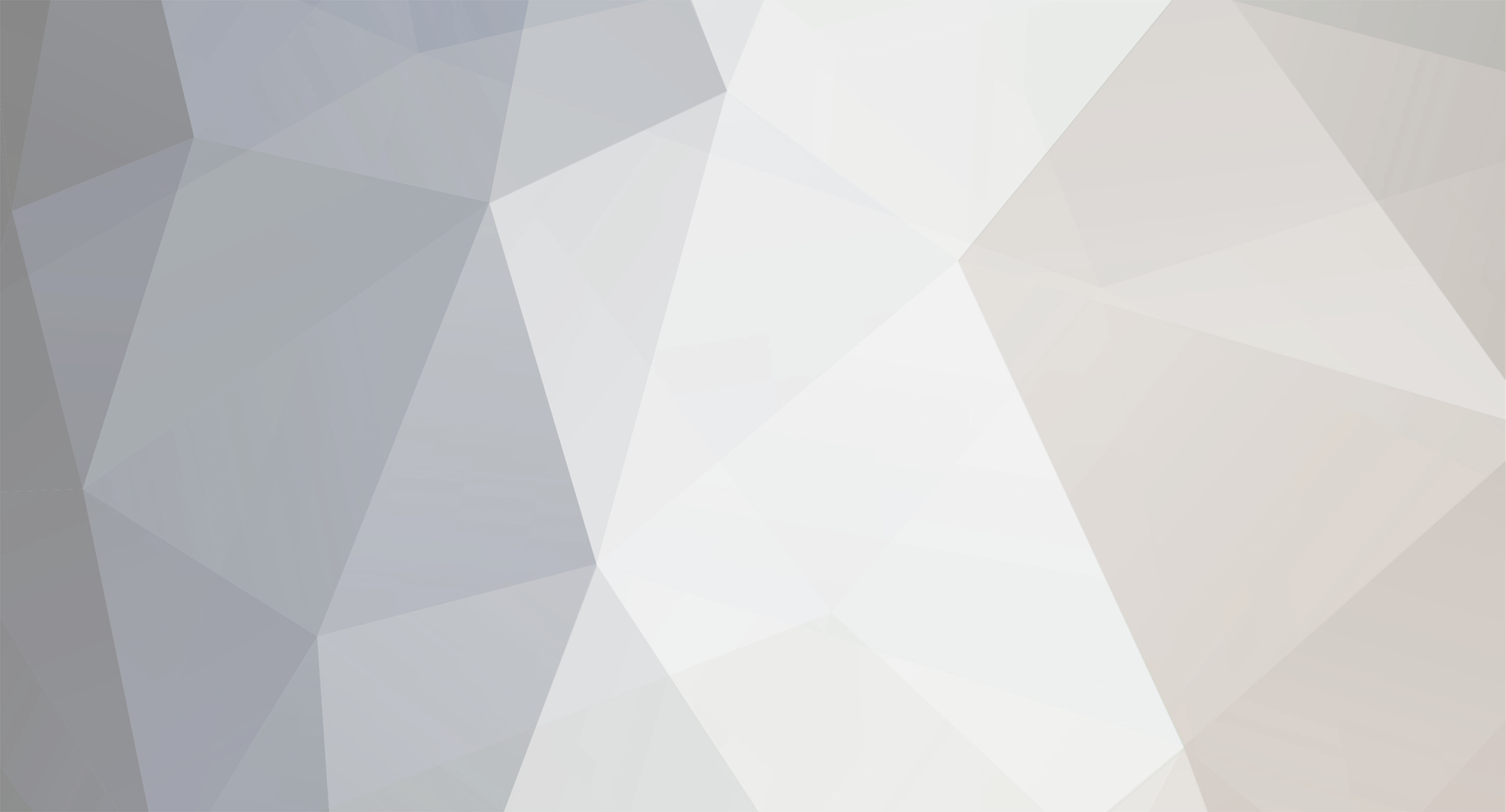
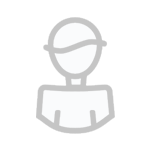
Leutech
-
Content Count
2 -
Joined
-
Last visited
Posts posted by Leutech
-
-
Greetings dear OC Community.
I have a problem with my script for a robot to farming an area.
The robot get the 'start farming' info from a other computer over modem_message.
Here is the code from the computer that sends the start farming info to the robot.
local component = require("component") local term = require("term") local event = require("event") local fs = require("filesystem") local gpu = component.gpu local sensor = component.sensor local modem = component.modem local port = 2154 local robot_modem = "d6d10a42-eda8-4ea9-bca2-ee06f3311622" local main_modem = "ad71011b-9016-4ad0-946f-76ee93d28d45" local X1, X2 = 2, 9 local Y1, Y2, Y3, Y4 = 0, 1, 2, 3 local Z1_1, Z1_2, Z2_1, Z2_2, Z3_1, Z3_2, Z4_1, Z4_2 = 0, 7, 8, 14, 15, 21, 22, 28 function scan_stages() count1 = 0 count2 = 0 count3 = 0 count4 = 0 print("Scanne nach Farm...") for x = X1,X2,1 do for z = Z1_1,Z1_2,1 do scan = sensor.scan(x, Y1, z) label = scan.block.label meta = scan.block.meta if ( label == "Barley" ) then if ( meta == 0.0 ) then count1 = count1 + 0 elseif ( meta == 1.0 ) then count1 = count1 + 1 elseif ( meta == 2.0 ) then count1 = count1 + 2 elseif ( meta == 3.0 ) then count1 = count1 + 3 end else count1 = count1 - 1 end end for z = Z2_1,Z2_2,1 do scan = sensor.scan(x, Y2, z) label = scan.block.label meta = scan.block.meta if ( label == "Barley" ) then if ( meta == 0.0 ) then count2 = count2 + 0 elseif ( meta == 1.0 ) then count2 = count2 + 1 elseif ( meta == 2.0 ) then count2 = count2 + 2 elseif ( meta == 3.0 ) then count2 = count2 + 3 end else count2 = count2 - 1 end end for z = Z3_1,Z3_2,1 do scan = sensor.scan(x, Y3, z) label = scan.block.label meta = scan.block.meta if ( label == "Barley" ) then if ( meta == 0.0 ) then count3 = count3 + 0 elseif ( meta == 1.0 ) then count3 = count3 + 1 elseif ( meta == 2.0 ) then count3 = count3 + 2 elseif ( meta == 3.0 ) then count3 = count3 + 3 end else count3 = count3 - 1 end end for z = Z4_1,Z4_2,1 do scan = sensor.scan(x, Y4, z) label = scan.block.label meta = scan.block.meta if ( label == "Barley" ) then if ( meta == 0.0 ) then count4 = count4 + 0 elseif ( meta == 1.0 ) then count4 = count4 + 1 elseif ( meta == 2.0 ) then count4 = count4 + 2 elseif ( meta == 3.0 ) then count4 = count4 + 3 end else count4 = count4 - 1 end end end totalCount = count1 + count2 + count3 + count4 send_info_to_robot() end function send_info_to_robot() if modem.isOpen(port) == false then modem.open(port) end modem.setStrength(400) print("Totale Anzal: " ..totalCount) if ( totalCount == 696 ) then print("Sende 'TRUE' Nachricht.") modem.send(robot_modem, port, "STARTING") elseif ( totalCount < 696 ) and ( totalCount > 0 ) then print("Sende 'FALSE' Nachricht.") modem.send(robot_modem, port, "WAITING") elseif ( totalCount < 0 ) then print("Sende 'TRUE' Nachricht.") modem.send(robot_modem, port, "STARTING") end local md, lA, rA, pt, distance, message = event.pull("modem_message") if message == "NOPOSITION" then print("ROBOTER HAT NICHT ENDPOSITION!") while true do local id, _ = event.pull("interrupted") computer.beep(1000, 10) if id == "interrupted" then break end end elseif message == "OK" then os.sleep(900) scan_stages() end end term.clear() scan_stages()
And here ist the farming code from the robot.
local component = require("component") local term = require("term") local event = require("event") local sides = require("sides") local r = require("robot") local rs = component.redstone local inv = component.inventory_controller local modem = component.modem local nav = component.navigation local robot_modem = "d6d10a42-eda8-4ea9-bca2-ee06f3311622" local server_modem = "1c0a3025-9ffc-43c1-9e03-39b6bca9b383" local big_stage = 64 local small_stage = 56 local start_posX = 226.5 local start_posY = 71.5 local start_posZ = -26.5 local port = 2154 function init_farming() if r.placeDown() == false then r.useDown() r.placeDown() else r.placeDown() end end function start_farming_route() r.select(1) for i = 33,1,-1 do r.forward() end for i = 4,1,-1 do r.down() end r.turnleft() farm_first_stage() end function farming() for i = 7,1,-1 do init_farming() r.forward() end init_farming() end function turi() r.turnRight() r.forward() r.turnRight() end function tule() r.turnLeft() r.forward() r.turnLeft() end function farm_first_stage() farming() tule() farming() turi() farming() tule() farming() turi() farming() tule() farming() turi() farming() tule() farming() r.up() turi() farm_second_stage() end function farm_second_stage() r.select(2) farming() tule() farming() turi() farming() tule() farming() turi() farming() tule() farming() turi() farming() r.up() tule() farm_third_stage() end function farm_third_stage() r.select(3) farming() turi() farming() tule() farming() turi() farming() tule() farming() turi() farming() tule() farming() r.up() turi() farm_fourth_stage() end function farm_fourth_stage() r.select(4) farming() tule() farming() turi() farming() tule() farming() turi() farming() tule() farming() turi() farming() r.up() r.turnLeft() r.forward() r.forward() r.turnLeft() for i = 7,1,-1 do r.forward() end r.turnLeft() r.back() r.back() wait_for_next() end function wait_for_next() os.sleep(10) get_info_from_main() end function check_current_slot(selectedSlot, slot, value) r.select(selectedSlot) if not slot then inv.suckFromSlot(3, selectedSlot, value) elseif slot.name == "harvestcraft:barleyitem" then count = slot.size if count < value then difference = value - count inv.suckFromSlot(3, selectedSlot, difference) end else r.turnAround() count = slot.size r.dropIntoSlot(3, selectedSlot, count) r.turnAround() inv.suckFromSlot(3, selectedSlot, value) end end function start_farming() local posX, posY, posZ = nav.getPosition() local facing = nav.getFacing() local slot1 = inv.getStackInInternalSlot(1) local slot2 = inv.getStackInInternalSlot(2) local slot3 = inv.getStackInInternalSlot(3) local slot4 = inv.getStackInInternalSlot(4) r.select(1) if ( posX == start_posX ) and ( posY == start_posY ) and ( posZ == start_posZ ) then modem.send(server_modem, port, "OK") else modem.send(server_modem, port, "NOPOSITION") end if (facing == 2.0 ) then r.turnLeft() elseif ( facing == 5.0 ) then r.turnAround() elseif ( facing == 3.0 ) then r.turnRight() end check_current_slot(1, slot1, big_stage) check_current_slot(2, slot2, small_stage) check_current_slot(3, slot3, small_stage) check_current_slot(4, slot4, small_stage) rs.setOutput(0, 20) os.sleep(120) rs.setOutput(0, 0) r.turnRight() start_farming_route() end function get_info_from_main() if modem.isOpen(port) == false then modem.open(port) end modem.setStrength(400) local cp, lA, rA, pt, dist, msg = event.pull("modem_message") if msg == "WAITING" then wait_for_next() elseif msg == "STARTING" then start_farming() end end term.clear() get_info_from_main()
So the problem is the first things he do it perfect, he get the items in his inventory, send the rs output signal to farm the area and let the water go away after 2 minutes. But if he want to plant the items he get an error.
The error is this:
/home/Farming.lua:197: attempt to call a nil value (field 'select'): stack traceback: /home/Farming.lua:197: in global 'start_farming' /home/Farming.lua:233: in global 'get_info_from_main' /home/Farming.lua:237: in main chunk (...tail calls...) [C]: in function 'xpcall' machine:798: in global 'xpcall' /lib/process.lua:63: in function </lib/process.lua:59>/home/Farming.lua:189: attempt to index a nil value (global 'robot'): stack traceback: /home/Farming.lua:189: in global 'start_farming' /home/Farming.lua:225: in global 'get_info_from_main' /home/Farming.lua:229: in main chunk (...tail calls...) [C]: in function 'xpcall' machine:798: in global 'xpcall' /lib/process.lua:63: in function </lib/process.lua:59>/home/Farming.lua:43: attempt to call a nil value (field 'turnleft'): stack traceback: /home/Farming.lua:43: in global 'start_farming_route' /home/Farming.lua:210: in global 'start_farming' /home/Farming.lua:225: in global 'get_info_from_main' /home/Farming.lua:229: in main chunk (...tail calls...) [C]: in function 'xpcall' machine:798: in global 'xpcall' /lib/process.lua:63: in function </lib/process.lua:59>/home/Farming.lua:43: attempt to call a nil value (field 'turnleft'): stack traceback: /home/Farming.lua:43: in global 'start_farming_route' /home/Farming.lua:210: in global 'start_farming' /home/Farming.lua:225: in global 'get_info_from_main' /home/Farming.lua:229: in main chunk (...tail calls...) [C]: in function 'xpcall' machine:798: in global 'xpcall' /lib/process.lua:63: in function </lib/process.lua:59>/home/Farming.lua:43: attempt to call a nil value (field 'turnleft'): stack traceback: /home/Farming.lua:43: in global 'start_farming_route' /home/Farming.lua:210: in global 'start_farming' /home/Farming.lua:225: in global 'get_info_from_main' /home/Farming.lua:229: in main chunk (...tail calls...) [C]: in function 'xpcall' machine:798: in global 'xpcall' /lib/process.lua:63: in function </lib/process.lua:59>
Please can someone help me?
simple command "term.clear()" not working
in Programming
Posted
You need to import the term api with
local term = require("term")