- Sky
- Blueberry
- Slate
- Blackcurrant
- Watermelon
- Strawberry
- Orange
- Banana
- Apple
- Emerald
- Chocolate
- Charcoal
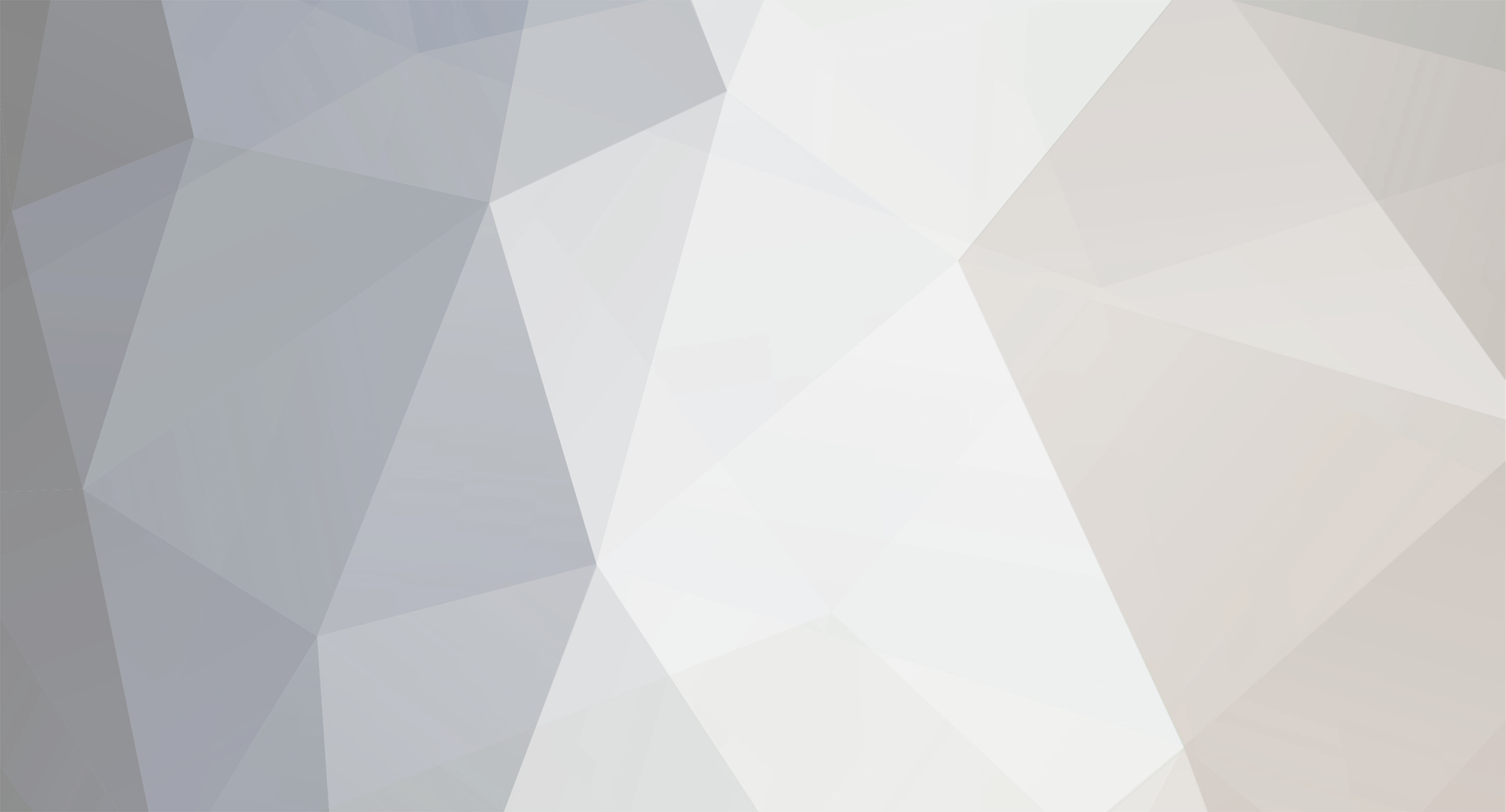
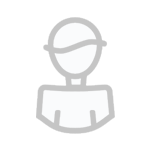
JuhaJGamer
-
Content Count
3 -
Joined
-
Last visited
-
Days Won
1
Posts posted by JuhaJGamer
-
-
I've been trying to implement an application to MineOS, an awesome Mac OS X Copy for OC.
This application is a clone of the Geoscan app that comes with the OS
I don't know why only one button of five in the GUI is responsive, but I do know that the responsive button is random, and changes each time the world is loaded.
All apps in MineOS use the ESCAPI API
local c = require("component") local event = require("event") local geo, holo local gpu = c.gpu local ecs = require("ECSAPI") local palette = require("palette") local computer = require("computer") local args = {...} --Проверка на наличие нужных уÑтройÑтв if not c.isAvailable("geolyzer") or not c.isAvailable("hologram") then ecs.error("This app needs a geolyzer and a Tier 2 Hologram Projector") return else geo = c.geolyzer holo = c.hologram end ------------------------- local sx, sz = 64, 64 local ox, oz = -32, -32 local starty, stopy = -10,10 local xSize, ySize = gpu.getResolution() local yCenter = math.floor(ySize / 2) --------------------------------------- local function getMemory() local totalMemory = computer.totalMemory() / 1024 local freeMemory = computer.freeMemory() / 1024 local usedMemory = totalMemory - freeMemory local stro4ka = math.ceil(usedMemory).."/"..math.floor(totalMemory).."KB" totalMemory, freeMemory, usedMemory = nil, nil, nil return stro4ka end local function changeScale() if currentScale < countOfScales then currentScale = currentScale + 1 else currentScale = 1 end holo.setScale(scales[currentScale]) end local function displayRow(x, yModifyer, z, tablica) local color for i = 1, #tablica do massiv[x][z][i] = math.ceil(massiv[x][z][i]) if tablica[i] > 0 then color = 1 if tablica[i] > 4 then color = 2 end if tablica[i + yModifyer] then holo.set(xScanTo - x + 1, i + yModifyer, zScanTo - z + 1, color) end end end color = nil tablica = nil end local function displayAllRows() clear() for x, val in pairs(massiv) do for z, val2 in pairs(massiv[x]) do displayRow(x, yModifyer, z, val2) end end end local function scan() ecs.clearScreen(0x33DBFF) component.hologram.clear() local barWidth = math.floor(xSize / 3 * 2) local xBar, yBar = math.floor(xSize/2 - barWidth / 2), yCenter local percent = 0 local countOfAll = (math.abs(sx) + math.abs(ox * 2) + 1) ^ 2 local counter = 0 for x=ox,sx+ox do for z=oz,sz+oz do local hx, hz = 1 + x - ox, 1 + z - oz local column = component.geolyzer.scan(x, z, false) for y=1,1+stopy-starty do local color = 0 if column then local hardness = column[y + starty + 32] if hardness == 0 or not hardness then color = 0 elseif hardness < 3 then color = 1 elseif hardness < 100 then color = 2 else color = 3 end end if component.hologram.maxDepth() > 1 then component.hologram.set(hx, y, hz, color) else component.hologram.set(hx, y, hz, math.min(color, 1)) end end percent = counter / countOfAll * 100 ecs.progressBar(xBar, yBar, barWidth, 1, 0xcccccc, ecs.colors.blue, percent) gpu.setForeground(0x444444) gpu.setBackground(0x33DBFF) ecs.centerText("x", yBar + 3, " "..math.floor(percent).."% Completed ") ecs.centerText("x", yBar + 2, " "..getMemory().." RAM ") counter = counter + 1 os.sleep(0) end end end local obj = {} local function newObj(class, name, ...) obj[class] = obj[class] or {} obj[class][name] = {...} end local currentHoloColor = ecs.colors.lime local function changeColorTo(color) currentHoloColor = color holo.setPaletteColor(1, color) holo.setPaletteColor(2, 0xffffff - color) end local function main() ecs.clearScreen(0x33DBFF) local yPos = yCenter - 14 ecs.clearScreen(0x33DBFF) newObj("buttons", " Start Scan ", ecs.drawAdaptiveButton("auto", yPos, 3, 1, " Start Scan ", 0x444444, 0xffffff)); yPos = yPos + 4 newObj("buttons", " Clear Scan ", ecs.drawAdaptiveButton("auto", yPos, 3, 1, " Clear Scan ", 0x444444, 0xffffff)); yPos = yPos + 4 newObj("buttons", " Y + 5 ", ecs.drawAdaptiveButton("auto", yPos, 3, 1, " Y + 5 ", 0x444444, 0xffffff)); yPos = yPos + 4 newObj("buttons", " Y - 5 ", ecs.drawAdaptiveButton("auto", yPos, 3, 1, " Y - 5 ", 0x444444, 0xffffff)); yPos = yPos + 4 newObj("buttons", " Button ", ecs.drawAdaptiveButton("auto", yPos, 3, 1, " Button ", 0x444444, 0xffffff)); yPos = yPos + 4 newObj("buttons", " Button ", ecs.drawAdaptiveButton("auto", yPos, 3, 1, " Button ", 0x444444, 0xffffff)); yPos = yPos + 4 newObj("buttons", " Exit ", ecs.drawAdaptiveButton("auto", yPos, 3, 1, " Exit ", 0x444444, 0xffffff)); yPos = yPos + 4 gpu.setBackground(0x33DBFF) gpu.setForeground(0x444444) ecs.centerText("x", yPos, "Y Range: "..stopy) end ---------------------------- main() while true do local e = {event.pull()} if e[1] == "touch" then for key, val in pairs(obj["buttons"]) do if ecs.clickedAtArea(e[3], e[4], obj["buttons"][key][1], obj["buttons"][key][2], obj["buttons"][key][3], obj["buttons"][key][4]) then ecs.drawAdaptiveButton(obj["buttons"][key][1], obj["buttons"][key][2], 3, 1, key, ecs.colors.green, 0xffffff) os.sleep(0.3) if key == " Start Scan " then scan() end if key == " Clear Scan " then holo.clear() end if key == " Y + 5 " then stopy = stopy + 5 starty = starty - 5 end if key == " Y - 5 " then stopy = stopy - 5 starty = starty + 5 end if key == " Exit " then ecs.prepareToExit() return 0 end end main() break end end end
-
Haha, it's nice to see this code, I think its great. Scrolling trough the 3DPrinter code, First thing that catches my eye :
drawingZoneCYKA = 0xCCCCCC
Dont worry, just translating
Help with colors in an Lua program
in Programming
Posted
I have no idea how to implement color mid-print but color is achieved by these commands
EDIT: Remember that you have to do the gpu getting at the first lines of the program(before anything else happens in the code)