- Sky
- Blueberry
- Slate
- Blackcurrant
- Watermelon
- Strawberry
- Orange
- Banana
- Apple
- Emerald
- Chocolate
- Charcoal
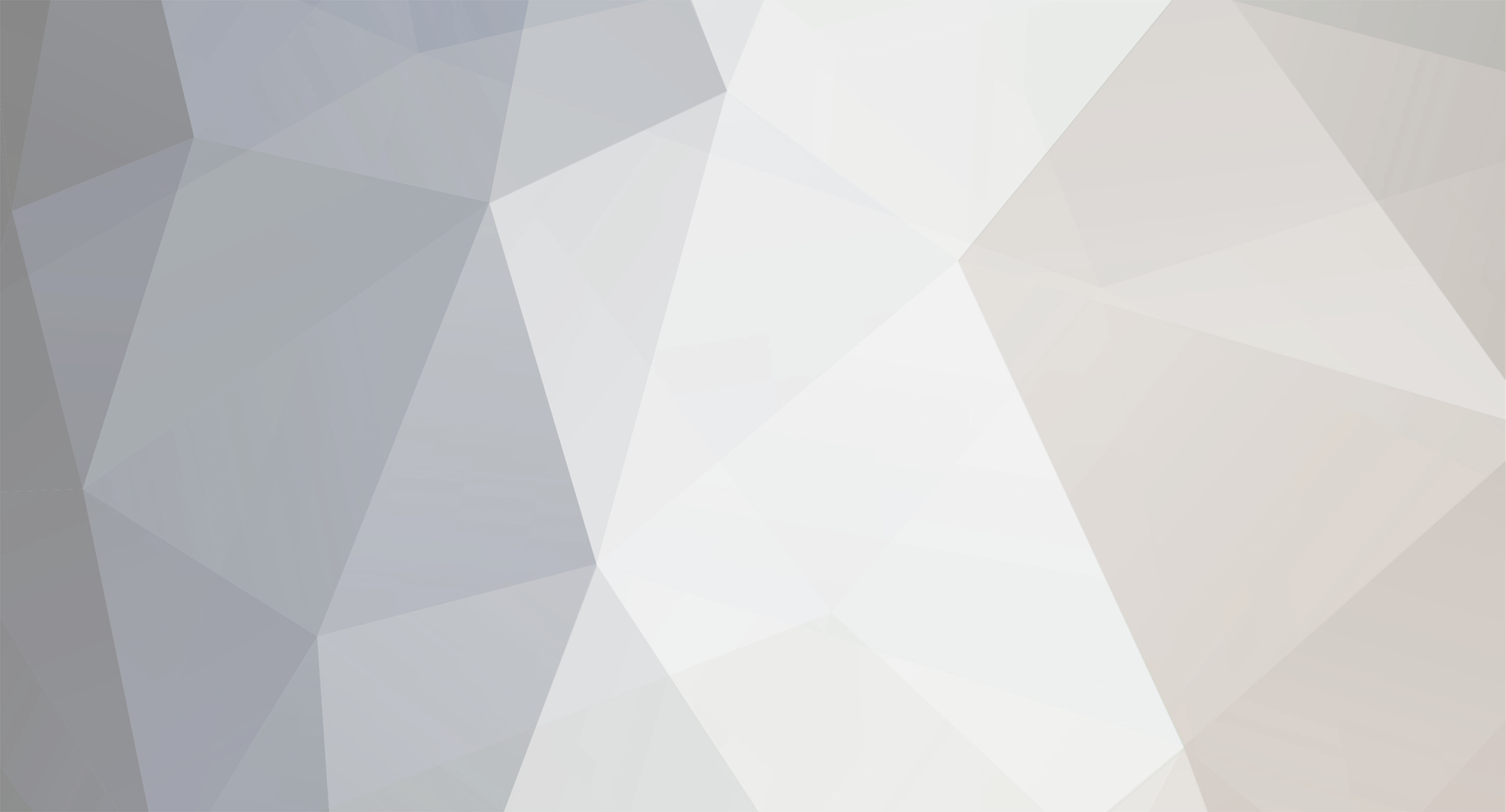
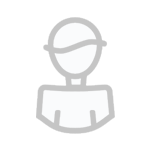
dieselfuelonly
-
Content Count
2 -
Joined
-
Last visited
Posts posted by dieselfuelonly
-
-
So I've been trying to write a program that can find unstackable/limited-stackability items within my AE2 network and export them to a different location. From a lot of playing around with adapter blocks and looking the the documentation when connected to various AE2 components I've found that I can use getItemsInNetwork() function when connecting an Adapter Block to an ME Controller on a network.
local component = require("component") local ser = require("serialization") contents = component.proxy(component.list("mekanism")()).getItemsInNetwork() for _,v in pairs(contents) do if not tonumber(v) then if v.hasTag or v.maxSize < 16 then print(v.name) end end end file = io.open("contents", "w") file:write(ser.serialize(contents)) file:close()
The little program above finds items with NBT tags or a stack size of less than 16, and prints out the names of these items. I was writing the table to a file as well just so I could have a better understanding of what information was contained within the item tables.
And yes, for some odd reason the ME Controller is referenced as a Mekanism block when connected to the Adapter block... lol. No idea why.
Now, comes the part I'm stuck at, and which appears may not be possible - but maybe I'm just missing something:
In order to move items I would need to use an adapter block connected to an ME export bus. The function used to set the configuration of the ME export bus needs a database entry containing the item I want the export.
The only way that I can find to write information to a database is by using the Inventory Controller module. And it appears that the store() function needs physical access to the item (whether in a robot slot, or in an inventory slot connected through an adapter/inv. controller when using a computer) in order to be able to create the database entry. Since I'm only able to pull a table with information about the items from the ME network, but not physically access the items to be able to use the store() function on, I can't seem to get anywhere.
Am I missing something or is it just not possible to do what I'm trying to accomplish?
Need help finding different ways of measuring RF usage
in Programming
Posted
I need some ideas on measuring RF usage.
A little background - I'm using Rotarycraft for power generation and am using OC to control engine control units connected to the engines themselves. The ECU lets me throttle the engine in 4 stages - 1/16th, 1/4, 1/2, and full power.
I have two engines powering my AE network - I use an adapter connected to the ME controller along with the getAvgPowerUsage() function to get the energy use of the AE network, then the program throttles the engines accordingly depending on how much power is needed to run the network - it works great, with the program selecting a throttle setting that produces the most minimal excess of power generation possible.
I'm trying to do something similar with RF. I'm using the RF Battery from ElectriCraft which has only two real useful functions that return the current RF stored in the battery, and the maximum amount of RF stored in the battery.
The problem I'm running into is how to accurately measure the amount of RF being used and adjusting the engines accordingly. Right now my program (messy and incomplete, see bottom) reads the change in stored energy in the battery every 5 seconds, and then attempts to adjust the throttles on the engine to produce an output value closer to keeping the battery at a just above "neutral" state.
What happens is it gets stuck in the cycle of producing too much power, throttling way down, sensing it's not producing enough power, returning the engines to maximum power, and repeating over and over. I've tried waiting for longer periods of time before changing the throttles on the engines, but this doesn't seem to smooth things out much. It's especially exaggerated because the engines don't immediately change from one power state to the other, instead they will smoothly wind up or down to reach the set power state.
The only other idea that I've had would be to find a way to cut the power input to the battery completely, then take two measurements 5 seconds apart to determine the power running out of the battery, and THEN set the engines to produce a slight excess of power. I can see this working, as it basically works just like my AE power program, where it has a defined energy target to produce and selects what ever throttle levels let it meet or exceed that target. However, it would be messy and a waste of very expensive ways to do that, so I was wondering if there were some other ideas?
Thanks