- Sky
- Blueberry
- Slate
- Blackcurrant
- Watermelon
- Strawberry
- Orange
- Banana
- Apple
- Emerald
- Chocolate
- Charcoal
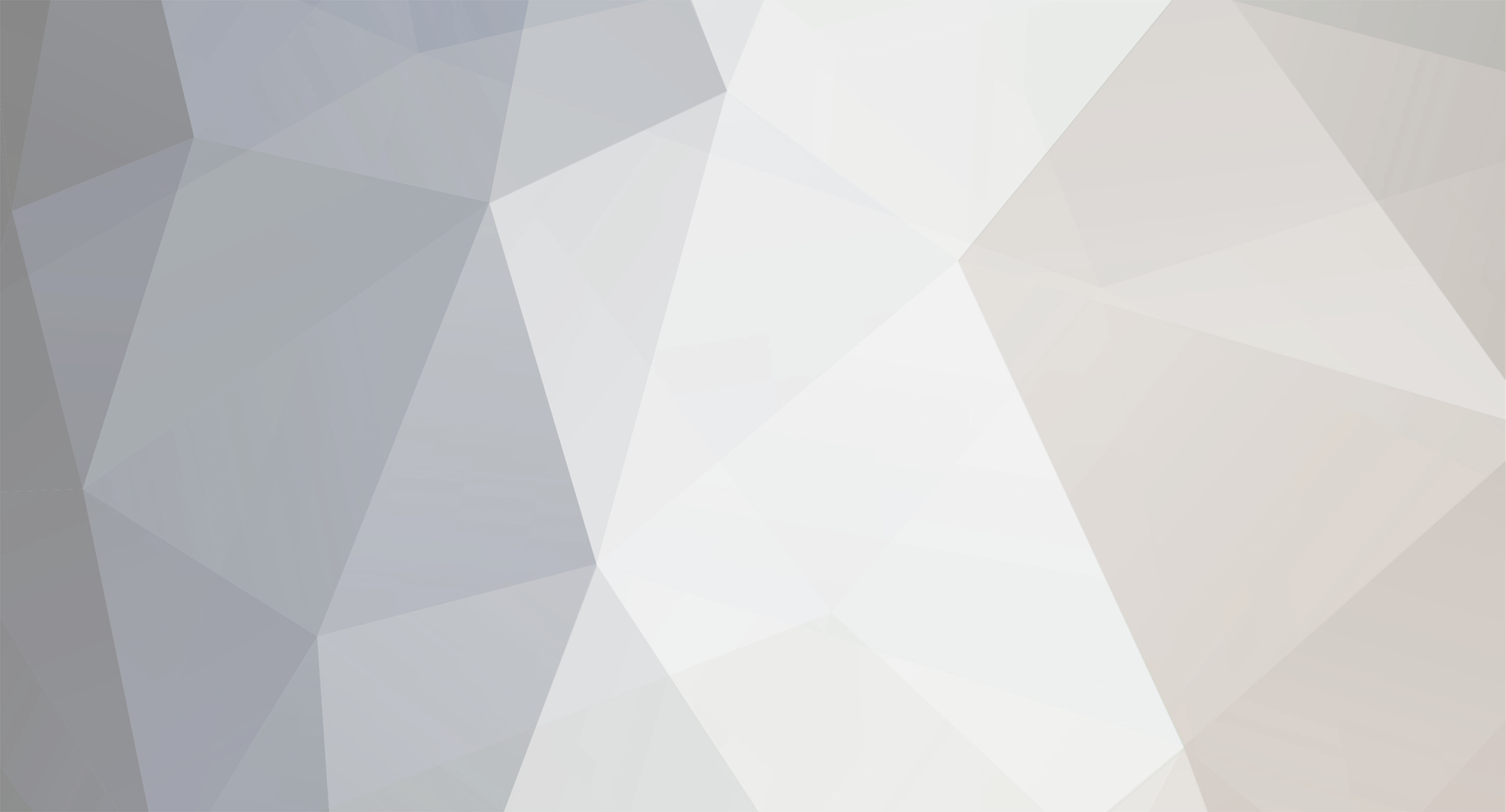
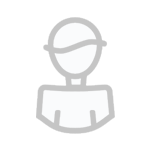
Karasuro
-
Content Count
7 -
Joined
-
Last visited
Posts posted by Karasuro
-
-
So I have tables in a text file. 1 line per table so that when I read from the file using var:lines() and put each line into a table in the program, they are accessible. However, now I have a table of strings that looks like this:
"{user = "ADMIN", pass = "ADMIN", settings = {}, admin = true}," "{user = "KARASURO", pass = "TEST", settings = {} }"
What would be the best way to get these tables from the file without them being strings?
(How does one table a string?)
-
Well, it works! it has a few graphical glitches and is a little slow even with a maxed creative build but it works.
Requirements:
Touch compatible screen
384K+ RAM
Any graphics card will work.
Tier 2+ CPU or APU recommended.
Controls:
R = Reset Simulation..
Space = Pause/Play simulation.
Up/Down arrows = Increase/Decrease Speed.
Left/Right arrows = Increase/Decrease Grid Size.Backspace = Exit Simulation.
WARNING!!
Due to graphical glitching, rapid screen flashing WILL occur. If you suffer from epilepsy, DO NOT RUN THIS PROGRAM.
Viewer Discretion is Advised.
Code:--[[ OpenLife v0.1.0 by Karasuro]] --[[ Imports ]] local component = require 'component' local gpu = component.gpu local term = require 'term' local event = require 'event' local keyboard = require 'keyboard' --[[*************************************** DECLARATIONS *******************************************]] local WIDTH, HEIGHT = gpu.getResolution() local size = 1 local pause = true local minDelay = 0.015625 local maxDelay = 0.125 local delay = maxDelay local timer = minDelay / 2 local cSize = 1 local screenTier = 1 local grid1 = {} local myEventHandlers = setmetatable({}, {__index = function() return unknownEvent end}) --[[********************************************** INITIALIZATION **************************************************]] gpu.setBackground(0x000000) gpu.setForeground(0xFFFFFF) term.clear() term.setCursorBlink(false) term.setCursor(1, 1) if (HEIGHT == 16) then screenTier = 1 elseif (HEIGHT == 25) then screenTier = 2 elseif (HEIGHT == 50) then screenTier = 3 end --[[********************************************************* PROGRAM METHODS *************************************************************]] -- Unknown Event Handler function unknownEvent() -- Do Nothing end -- Creating the Grid function setupGrid() if (screenTier == 1) then -- 50, 16 if (size <= 1) then cSize = 2 elseif (size >= 2) then cSize = 1 end elseif (screenTier == 2) then -- 80, 25 if (size <= 1) then cSize = 5 elseif (size >= 2) then cSize = 1 end elseif (screenTier == 3) then -- 160, 50 if (size <= 1) then cSize = 10 elseif (size == 2) then cSize = 5 elseif (size >= 3) then cSize = 2 end end grid1 = { width = WIDTH / cSize, height = HEIGHT / cSize} for x = 1, grid1.width do grid1[x] = {} for y = 1, grid1.height do grid1[x][y] = 0 end end end -- Touch Event Handler function myEventHandlers.touch(screenAdress, x, y, button, playerName) if (button == 0) then local i = math.ceil(x / cSize) local j = math.ceil(y / cSize) if (grid1[i][j] == 0) then grid1[i][j] = 1 else grid1[i][j] = 0 end end end -- Keyboard Event Handler function myEventHandlers.key_up(adress, char, code, playerName) -- Space Bar (Pause/Play) if (code == 0x39) then pause = not pause end -- Backspace (Exit Program) if (code == 0x0E) then gpu.setResolution(WIDTH, HEIGHT) gpu.setBackground(0x000000) gpu.setForeground(0xFFFFFF) term.clear() os.exit() end -- R key (Reset) if (code == 0x13) then pause = true delay = minDelay setupGrid() end -- Up key if (code == 0xC8) then if (delay > minDelay) then delay = delay / 2 end end -- Down key if (code == 0xD0) then if (delay < maxDelay) then delay = delay * 2 end end -- Right key if (code == 0xCD) then pause = true if (size < 5) then size = size + 1 end setupGrid() end -- Left key if (code == 0xCB) then Pause = true if (size > 1) then size = size - 1 end setupGrid() end end -- Event Handler Method function handleEvent(eventID, ...) if (eventID) then myEventHandlers[eventID](...) end end -- Cell Check Method function checkCells(x, y, g) local n = 0 for i = x-1, x+1 do for j = y-1, y+1 do if not (i < 1 or j < 1 or i > g.width or j > g.height or (i == x and j == y)) then n = n + g[i][j] end end end return n end setupGrid() --[[**************************************************************** MAIN LOOP ********************************************************************]] while true do --[[ UPDATE ]] if not pause then timer = timer * 2 if (timer >= delay) then timer = minDelay / 2 local grid2 = { width = WIDTH / cSize, height = HEIGHT / cSize } for x = 1, grid1.width do grid2[x] = {} local r = grid1[x] for y = 1, grid1.height do local n = checkCells(x, y, grid1) local l = r[y] grid2[x][y] = l if (n < 2) then grid2[x][y] = 0 elseif (n == 2) then grid2[x][y] = grid1[x][y] elseif (n == 3) then grid2[x][y] = 1 elseif (n > 3) then grid2[x][y] = 0 end end end grid1 = grid2 end end --[[ DRAW ]] gpu.setBackground(0x000000) gpu.setForeground(0xFFFFFF) term.clear() for x = 1, grid1.width do for y = 1, grid1.height do local i = (x*cSize)-(cSize-1) local j = (y*cSize)-(cSize-1) if (grid1[x][y] == 1) then gpu.setBackground(0xFFFFFF) else gpu.setBackground(0x000000) end gpu.fill(i, j, i+cSize, j+cSize, " ") end end handleEvent(event.pull(0.001)) end
-
I changed it so it won't use Elses at all, instead it runs through each IF then just returns TRUE.
local function checkPlayerPos(dir) if (dir == "up") and (player.y == 1) then return false end if (dir == "down") and (player.y == sH) then return false end if (dir == "left") and (player.x == 1) then return false end if (dir == "right") and (player.x == sW) then return false end return true end
-
So, I'm going to try and make a rogue-like dungeon crawler but I seem to have an issue with screen locking.
Basically, when you press an arrow key, the event handler runs the function below, through an if statement, and if true moves the player in that direction. sH and sW are values from gpu.getResolution(). However, if it is false then it should just skip the if statement entirely. Or am I wrong?
local function checkPlayerPos(dir) if (dir == "up") and (player.y == 1) then return false else return true end if (dir == "down") and (player.y == sH) then return false else return true end if (dir == "left") and (player.x == 1) then return false else return true end if (dir == "right") and (player.x == sW) then return false else return true end end
Entire Program:
--[[GameTest by Karasuro]]-- -- Requirements local comp = require "component"; local event = require "event"; local gpu = comp.gpu; local term = require "term"; local sW, sH = gpu.getResolution(); local player = {x=sW/2,y=sH/2,w=1,h=1,col={bg=0xAAAAAA,fg=0x00AA00}}; local running = false; -- Render Screen -- local function render() gpu.setBackground(0x000000); gpu.setForeground(0xFFFFFF); term.clear(); -- Render Player gpu.setBackground(player.col.bg); gpu.setForeground(player.col.fg); gpu.fill(player.x,player.y,player.w,player.h," "); gpu.setBackground(0x000000); gpu.setForeground(0xFFFFFF); end local function checkPlayerPos(dir) if (dir == "up") and (player.y == 1) then return false else return true end if (dir == "down") and (player.y == sH) then return false else return true end if (dir == "left") and (player.x == 1) then return false else return true end if (dir == "right") and (player.x == sW) then return false else return true end end --Event Handler local function wait() --Wait 1 cycle end local myEventHandlers = setmetatable({}, {__index = function() return wait end}); function myEventHandlers.key_up(adress, char, code, playerName) if (code == 0xC8) then if (checkPlayerPos("up")) then player.y = player.y - 1; render(); end end if (code == 0xD0) then if (checkPlayerPos("down")) then player.y = player.y + 1; render(); end end if (code == 0xCB) then if (checkPlayerPos("left")) then player.x = player.x - 1; render(); end end if (code == 0xCD) then if (checkPlayerPos("right")) then player.x = player.x + 1; render(); end end end local function handleEvent(eventID, ...) if (eventID) then myEventHandlers[eventID](...); end end if (running == false) then running = true; render(); end while running do handleEvent(event.pull(0.001)); end
-
Yeah, I don't know why I went in and added the [""] to the tables. I guess I wasn't really thinking at the time. XP Brain process was string instead of variable.
Also, I was looking for a better way to make the buttons array. That helps me out a lot!
However, I'll need to figure out an algorith for pitch.. the scale is F# Major (F#3 - F#5)
This may also allow me to implement a setScale() function and instead of using note blocks just use computer.beep(). Larger range and note durration support. ;P
The original design had speed variation hence the tempo and dt variables.
Also I need to figure out if doing multiple note.trigger() at once is possible..
This project is a port from my Love2D application AudynMatrix.
https://dl.dropboxusercontent.com/u/16913227/AudynMatrix.zip
-
Hello! Karasuro here. I just downloaded OpenComputers recently and have been testing things out. Here is my first program I've written in OC and it needs lots of improvement and optimization but at least it works.
Note:
You may need a high-end computer for this to work. I made it using Teir 3 everything. (Creative Mode)
Also you will need to hook up a note block.Now checks the resolution of the system before changing it's local resolution. Returns to the previous resolution upon exit.
To-Do:
Add variable tempo.
Switch to Computer.beep() for extra pitch support.
Add variable scales.
Code: Optimized to mpmxyz's recommendations.--[[OpenMatrix v0.7.1 by Karasuro]]-- -- Requirements local computer = require "computer"; local component = require "component"; local term = require "term"; local event = require "event"; local gpu = component.gpu; local source = component.note_block; local note = require "note"; local keyboard = require "keyboard"; local color = require "colors"; -- Variables and Tables term.clear(); local maxX, maxY = gpu.getResolution(); if (maxX ~= 80) then if (maxY ~= 25) then gpu.setResolution(80, 25); end end local w, h = gpu.getResolution(); local UI = {bg=0x000000, fg=0xFFFFFF, txt=0x000000, width=w, height=h}; local UI_btn = {on=0xFFFFFF, off=0xFFAA00, play=0xFFFF55}; local UI_menu = {default=0xFFAA00, hit=0xFFFF55}; local buttons = {}; for Y = 2,16 do local row = {}; table.insert(buttons, row); for X = 2,47,3 do if (Y == 2) then table.insert(row, {state=3, posX=X, posY=Y, pitch=25}); elseif (Y == 3) then table.insert(row, {state=3, posX=X, posY=Y, pitch=24}); elseif (Y == 4) then table.insert(row, {state=3, posX=X, posY=Y, pitch=22}); elseif (Y == 5) then table.insert(row, {state=3, posX=X, posY=Y, pitch=20}); elseif (Y == 6) then table.insert(row, {state=3, posX=X, posY=Y, pitch=18}); elseif (Y == 7) then table.insert(row, {state=3, posX=X, posY=Y, pitch=17}); elseif (Y == 8) then table.insert(row, {state=3, posX=X, posY=Y, pitch=15}); elseif (Y == 9) then table.insert(row, {state=3, posX=X, posY=Y, pitch=13}); elseif (Y == 10) then table.insert(row, {state=3, posX=X, posY=Y, pitch=12}); elseif (Y == 11) then table.insert(row, {state=3, posX=X, posY=Y, pitch=10}); elseif (Y == 12) then table.insert(row, {state=3, posX=X, posY=Y, pitch=8}); elseif (Y == 13) then table.insert(row, {state=3, posX=X, posY=Y, pitch=6}); elseif (Y == 14) then table.insert(row, {state=3, posX=X, posY=Y, pitch=5}); elseif (Y == 15) then table.insert(row, {state=3, posX=X, posY=Y, pitch=3}); else table.insert(row, {state=3, posX=X, posY=Y, pitch=1}) end end end local printDebug = false; local mX = 0; local mY = 0; local pitch = 1; local playing = false; local tick = 0; local beat = 16; local tempo = 40; local cycle = 0; local dt = 0; local menu = { {id="play", posX=2, posY=(h - 1), state=1, label="Play", width=6, height=1} }; local header = {posX=47,posY=18,width=2,height=1,col=0x00AA00}; local myEventHandlers = setmetatable({}, {__index = function() return unknownEvent end}); -- Functions function unknownEvent() -- Do Nothing! end function myEventHandlers.key_up(adress, char, code, playerName) -- Space Bar if (code == 0x39) then playing = not playing; end -- Backspace if (code == 0x0E) then gpu.setResolution(maxX, maxY); term.clear(); os.exit(); end -- Grave or ~ if (code == 0x29) then printDebug = not printDebug; end end function myEventHandlers.touch(screenAdress, x, y, button, playerName) mX = x; mY = y; if (button == 0) then -- Buttons Table Handling for r in pairs(buttons) do for c in pairs(buttons[r]) do if (mX >= buttons[r][c].posX) and (mX <= (buttons[r][c].posX + 2)) and (mY == buttons[r][c].posY) then if (buttons[r][c].state == 3) then buttons[r][c].state = 1; else buttons[r][c].state = 3; end end end end -- Menu Table Handling for i in pairs(menu) do if (mX >= menu[i].posX) and (mX <= (menu[i].posX + menu[i].width)) and (mY == menu[i].posY) then --play/stop button if menu[i].id == "play" then playing = not playing; menu[i].label = "Stop"; end end end end end function handleEvent(eventID, ...) if (eventID) then myEventHandlers[eventID](...); end end function playTone(pitch) if (source ~= nil) then source.trigger(pitch); else computer.beep(pitch); end end --Draw Instructions ONCE gpu.setBackground(UI.bg); --Black gpu.setForeground(UI.fg); --White gpu.set(2,20,"~ = debug info | space = play/stop | backspace = exit"); gpu.set(2,21,"Multiple notes on each column = more processing time.") -- Main Loop while true do --[[UPDATE DATA]]-- if playing then -- Update UI menu[1].label = "Stop"; -- Start ticking tick = tick + (tempo * 0.1); if tick > 1 then header.posX = header.posX + 3; if header.posX > 47 then header.posX = 2; end tick = 0; beat = beat + 1; end if (beat > 16) then beat = 1; end -- Get Active Notes for r in pairs(buttons) do for c in pairs(buttons[r]) do if (buttons[r][c].posX == header.posX) then if (buttons[r][c].state == 1) then buttons[r][c].state = 2; pitch = buttons[r][c].pitch; playTone(pitch); end else if (buttons[r][c].state == 2) then buttons[r][c].state = 1; end end end end else -- Reset UI header.posX = 47; tick = 0; beat = 16; menu[1].label = "Play"; for r in pairs(buttons) do for c in pairs(buttons[r]) do if (buttons[r][c].state == 2) or (buttons[r][c].state == 1) then buttons[r][c].state = 1; else buttons[r][c].state = 3; end end end end --[[DRAW STUFF]]-- -- Draw Buttons and their states for r in pairs(buttons) do for c in pairs(buttons[r]) do if (buttons[r][c].state == 3) then --Draw Button OFF gpu.setBackground(UI_btn.off); --Orange gpu.fill(buttons[r][c].posX,buttons[r][c].posY,2,1," "); gpu.setBackground(UI.bg); --Black end if (buttons[r][c].state == 2) then -- Draw Buttons ON gpu.setBackground(UI_btn.on); --White gpu.fill(buttons[r][c].posX,buttons[r][c].posY,2,1," "); gpu.setBackground(UI.bg); --Black end if (buttons[r][c].state == 1) then -- Draw Buttons PLAY gpu.setBackground(UI_btn.play); --Yellow gpu.fill(buttons[r][c].posX,buttons[r][c].posY,2,1," "); gpu.setBackground(UI.bg); --Black end end end -- Draw Play Head gpu.setBackground(header.col); --Green gpu.fill(header.posX,header.posY,2,1," "); gpu.setBackground(UI.bg); --Black if (header.posX ~= 2) then gpu.fill((header.posX - 3),header.posY,2,1," "); end if (header.posX == 2) then gpu.fill(47,18,2,1," "); end if (header.posX == 47) then gpu.fill(2,header.posY,45,1," "); end --Draw Menu for i in pairs(menu) do if (menu[i].state == 1) then gpu.setBackground(UI_menu.default) --Orange gpu.fill(menu[i].posX,menu[i].posY,menu[i].width,menu[i].height," "); gpu.setForeground(UI.txt); --Black gpu.set(menu[i].posX,menu[i].posY,menu[i].label); gpu.setBackground(UI.bg); --Black gpu.setForeground(UI.fg); --White end if (menu[i].state == 2) then gpu.setBackground(UI_menu.hit); --Yellow gpu.fill(menu[i].posX,menu[i].posY,menu[i].width,menu[i].height," "); gpu.setForeground(UI.txt); --Black gpu.set(menu[i].posX,menu[i].posY,menu[i].label); gpu.setBackground(UI.bg); --Black gpu.setForeground(UI.fg); --White end end -- print debug information if (printDebug) then gpu.fill(50,2,80,5," "); gpu.setBackground(UI.bg) --Black gpu.setForeground(UI.fg) --White gpu.set(50,2,tostring(mX)..", "..tostring(mY)); gpu.set(50,3,"playing: "..tostring(playing)); gpu.set(50,4,"beat: "..tostring(beat)); gpu.set(50,5,"pitch: "..tostring(pitch)); else gpu.fill(50,2,80,5," "); end handleEvent(event.pull(0.001)); end
io.read string to table
in Programming
Posted
I'm only receiving the last line from the file in my table so there's only 1 entry?